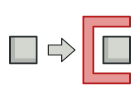
Procuration en Ruby
La Procuration est un patron de conception structurel qui fournit un objet qui agit comme un substitut pour un objet du service utilisé par un client. Une procuration reçoit les demandes d’un client, effectue des tâches (contrôle des accès, mise en cache, etc.) et passe ensuite la demande à un objet du service.
L’objet Procuration possède la même interface qu’un service, ce qui le rend interchangeable avec un vrai objet lorsqu’il est passé à un client.
Complexité :
Popularité :
Exemples d’utilisation : La procuration n’est pas souvent invitée en Ruby, mais elle se montre très pratique dans certains cas. Elle est incontournable lorsque vous voulez ajouter de nouveaux comportements à un objet d’une classe existante sans modifier le code client.
Identification : Les procurations délèguent tout le travail à un autre objet. Chaque méthode de la procuration devrait au final, faire référence à un objet du service, sauf si la procuration est une sous-classe d’un service.
Exemple conceptuel
Dans cet exemple, nous allons voir la structure de la Procuration. Nous allons répondre aux questions suivantes :
- Que contiennent les classes ?
- Quels rôles jouent-elles ?
- Comment les éléments du patron sont-ils reliés ?
main.rb: Exemple conceptuel
# The Subject interface declares common operations for both RealSubject and the
# Proxy. As long as the client works with RealSubject using this interface,
# you'll be able to pass it a proxy instead of a real subject.
class Subject
# @abstract
def request
raise NotImplementedError, "#{self.class} has not implemented method '#{__method__}'"
end
end
# The RealSubject contains some core business logic. Usually, RealSubjects are
# capable of doing some useful work which may also be very slow or sensitive -
# e.g. correcting input data. A Proxy can solve these issues without any changes
# to the RealSubject's code.
class RealSubject < Subject
def request
puts 'RealSubject: Handling request.'
end
end
# The Proxy has an interface identical to the RealSubject.
class Proxy < Subject
# @param [RealSubject] real_subject
def initialize(real_subject)
@real_subject = real_subject
end
# The most common applications of the Proxy pattern are lazy loading, caching,
# controlling the access, logging, etc. A Proxy can perform one of these
# things and then, depending on the result, pass the execution to the same
# method in a linked RealSubject object.
def request
return unless check_access
@real_subject.request
log_access
end
# @return [Boolean]
def check_access
puts 'Proxy: Checking access prior to firing a real request.'
true
end
def log_access
print 'Proxy: Logging the time of request.'
end
end
# The client code is supposed to work with all objects (both subjects and
# proxies) via the Subject interface in order to support both real subjects and
# proxies. In real life, however, clients mostly work with their real subjects
# directly. In this case, to implement the pattern more easily, you can extend
# your proxy from the real subject's class.
def client_code(subject)
# ...
subject.request
# ...
end
puts 'Client: Executing the client code with a real subject:'
real_subject = RealSubject.new
client_code(real_subject)
puts "\n"
puts 'Client: Executing the same client code with a proxy:'
proxy = Proxy.new(real_subject)
client_code(proxy)
output.txt: Résultat de l’exécution
Client: Executing the client code with a real subject:
RealSubject: Handling request.
Client: Executing the same client code with a proxy:
Proxy: Checking access prior to firing a real request.
RealSubject: Handling request.
Proxy: Logging the time of request.