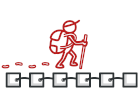
루비로 작성된 반복자
반복자는 복잡한 데이터 구조의 내부 세부 정보를 노출하지 않고 해당 구조를 차례대로 순회할 수 있도록 하는 행동 디자인 패턴입니다.
반복자 덕분에 클라이언트들은 단일 반복기 인터페이스를 사용하여 유사한 방식으로 다른 컬렉션들의 요소들을 탐색할 수 있습니다.
복잡도:
인기도:
사용 예시들: 이 패턴은 루비 코드에 자주 사용됩니다. 많은 프레임워크들과 라이브러리들은 이 패턴을 컬렉션을 탐색하는 표준 방법을 제공하기 위해 사용합니다.
식별법: 반복자는 그의 탐색 메서드들(예: next
, previous
등)로 쉽게 인식할 수 있습니다. 또 반복자를 사용하는 클라이언트 코드는 반복자가 순회하는 컬렉션을 직접 접근하지 못할 수도 있습니다.
개념적인 예시
이 예시는 반복자 디자인 패턴의 구조를 보여주고 다음 질문에 중점을 둡니다:
- 패턴은 어떤 클래스들로 구성되어 있나요?
- 이 클래스들은 어떤 역할을 하나요?
- 패턴의 요소들은 어떻게 서로 연관되어 있나요?
main.rb: 개념적인 예시
class AlphabeticalOrderIterator
# In Ruby, the Enumerable mixin provides classes with several traversal and
# searching methods, and with the ability to sort. The class must provide a
# method each, which yields successive members of the collection.
include Enumerable
# This attribute indicates the traversal direction.
attr_accessor :reverse
private :reverse
# @return [Array]
attr_accessor :collection
private :collection
# @param [Array] collection
# @param [Boolean] reverse
def initialize(collection, reverse: false)
@collection = collection
@reverse = reverse
end
def each(&block)
return @collection.reverse.each(&block) if reverse
@collection.each(&block)
end
end
class WordsCollection
# @return [Array]
attr_accessor :collection
private :collection
def initialize(collection = [])
@collection = collection
end
# The `iterator` method returns the iterator object itself, by default we
# return the iterator in ascending order.
def iterator
AlphabeticalOrderIterator.new(@collection)
end
# @return [AlphabeticalOrderIterator]
def reverse_iterator
AlphabeticalOrderIterator.new(@collection, reverse: true)
end
# @param [String] item
def add_item(item)
@collection << item
end
end
# The client code may or may not know about the Concrete Iterator or Collection
# classes, depending on the level of indirection you want to keep in your
# program.
collection = WordsCollection.new
collection.add_item('First')
collection.add_item('Second')
collection.add_item('Third')
puts 'Straight traversal:'
collection.iterator.each { |item| puts item }
puts "\n"
puts 'Reverse traversal:'
collection.reverse_iterator.each { |item| puts item }
output.txt: 실행 결과
Straight traversal:
First
Second
Third
Reverse traversal:
Third
Second
First