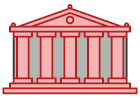
Facade em C#
O Facade é um padrão de projeto estrutural que fornece uma interface simplificada (mas limitada) para um sistema complexo de classes, biblioteca, ou framework.
Embora o Facade diminua a complexidade geral do aplicativo, também ajuda a mover dependências indesejadas para um só local.
Complexidade:
Popularidade:
Exemplos de uso: O padrão Facade é comumente usado em aplicações escritas em C#. É especialmente útil ao trabalhar com bibliotecas e APIs complexas.
Identificação: O Facade pode ser reconhecido em uma classe que possui uma interface simples, mas delega a maior parte do trabalho para outras classes. Geralmente, as fachadas gerenciam o ciclo de vida completo dos objetos que usam.
Exemplo conceitual
Este exemplo ilustra a estrutura do padrão de projeto Facade. Ele se concentra em responder a estas perguntas:
- De quais classes ele consiste?
- Quais papéis essas classes desempenham?
- De que maneira os elementos do padrão estão relacionados?
Program.cs: Exemplo conceitual
using System;
namespace RefactoringGuru.DesignPatterns.Facade.Conceptual
{
// The Facade class provides a simple interface to the complex logic of one
// or several subsystems. The Facade delegates the client requests to the
// appropriate objects within the subsystem. The Facade is also responsible
// for managing their lifecycle. All of this shields the client from the
// undesired complexity of the subsystem.
public class Facade
{
protected Subsystem1 _subsystem1;
protected Subsystem2 _subsystem2;
public Facade(Subsystem1 subsystem1, Subsystem2 subsystem2)
{
this._subsystem1 = subsystem1;
this._subsystem2 = subsystem2;
}
// The Facade's methods are convenient shortcuts to the sophisticated
// functionality of the subsystems. However, clients get only to a
// fraction of a subsystem's capabilities.
public string Operation()
{
string result = "Facade initializes subsystems:\n";
result += this._subsystem1.operation1();
result += this._subsystem2.operation1();
result += "Facade orders subsystems to perform the action:\n";
result += this._subsystem1.operationN();
result += this._subsystem2.operationZ();
return result;
}
}
// The Subsystem can accept requests either from the facade or client
// directly. In any case, to the Subsystem, the Facade is yet another
// client, and it's not a part of the Subsystem.
public class Subsystem1
{
public string operation1()
{
return "Subsystem1: Ready!\n";
}
public string operationN()
{
return "Subsystem1: Go!\n";
}
}
// Some facades can work with multiple subsystems at the same time.
public class Subsystem2
{
public string operation1()
{
return "Subsystem2: Get ready!\n";
}
public string operationZ()
{
return "Subsystem2: Fire!\n";
}
}
class Client
{
// The client code works with complex subsystems through a simple
// interface provided by the Facade. When a facade manages the lifecycle
// of the subsystem, the client might not even know about the existence
// of the subsystem. This approach lets you keep the complexity under
// control.
public static void ClientCode(Facade facade)
{
Console.Write(facade.Operation());
}
}
class Program
{
static void Main(string[] args)
{
// The client code may have some of the subsystem's objects already
// created. In this case, it might be worthwhile to initialize the
// Facade with these objects instead of letting the Facade create
// new instances.
Subsystem1 subsystem1 = new Subsystem1();
Subsystem2 subsystem2 = new Subsystem2();
Facade facade = new Facade(subsystem1, subsystem2);
Client.ClientCode(facade);
}
}
}
Output.txt: Resultados da execução
Facade initializes subsystems:
Subsystem1: Ready!
Subsystem2: Get ready!
Facade orders subsystems to perform the action:
Subsystem1: Go!
Subsystem2: Fire!