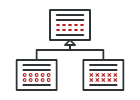
러스트로 작성된 템플릿 메서드
템플릿 메서드는 기초 클래스에서 알고리즘의 골격을 정의할 수 있도록 하는 행동 디자인 패턴입니다. 또 이 패턴은 자식 클래스들이 전체 알고리즘의 구조를 변경하지 않고도 기본 알고리즘의 단계들을 오버라이드할 수 있도록 합니다.
Conceptual Example
main.rs
trait TemplateMethod {
fn template_method(&self) {
self.base_operation1();
self.required_operations1();
self.base_operation2();
self.hook1();
self.required_operations2();
self.base_operation3();
self.hook2();
}
fn base_operation1(&self) {
println!("TemplateMethod says: I am doing the bulk of the work");
}
fn base_operation2(&self) {
println!("TemplateMethod says: But I let subclasses override some operations");
}
fn base_operation3(&self) {
println!("TemplateMethod says: But I am doing the bulk of the work anyway");
}
fn hook1(&self) {}
fn hook2(&self) {}
fn required_operations1(&self);
fn required_operations2(&self);
}
struct ConcreteStruct1;
impl TemplateMethod for ConcreteStruct1 {
fn required_operations1(&self) {
println!("ConcreteStruct1 says: Implemented Operation1")
}
fn required_operations2(&self) {
println!("ConcreteStruct1 says: Implemented Operation2")
}
}
struct ConcreteStruct2;
impl TemplateMethod for ConcreteStruct2 {
fn required_operations1(&self) {
println!("ConcreteStruct2 says: Implemented Operation1")
}
fn required_operations2(&self) {
println!("ConcreteStruct2 says: Implemented Operation2")
}
}
fn client_code(concrete: impl TemplateMethod) {
concrete.template_method()
}
fn main() {
println!("Same client code can work with different concrete implementations:");
client_code(ConcreteStruct1);
println!();
println!("Same client code can work with different concrete implementations:");
client_code(ConcreteStruct2);
}
Output
Same client code can work with different concrete implementations: TemplateMethod says: I am doing the bulk of the work ConcreteStruct1 says: Implemented Operation1 TemplateMethod says: But I let subclasses override some operations ConcreteStruct1 says: Implemented Operation2 TemplateMethod says: But I am doing the bulk of the work anyway Same client code can work with different concrete implementations: TemplateMethod says: I am doing the bulk of the work ConcreteStruct2 says: Implemented Operation1 TemplateMethod says: But I let subclasses override some operations ConcreteStruct2 says: Implemented Operation2 TemplateMethod says: But I am doing the bulk of the work anyway