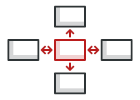
Mediator en Go
Mediator es un patrón de diseño de comportamiento que reduce el acoplamiento entre los componentes de un programa haciendo que se comuniquen indirectamente a través de un objeto mediador especial.
El patrón Mediator facilita la modificación, extensión y reutilización de componentes individuales porque ya no son dependientes de todas las demás clases.
Ejemplo conceptual
Un ejemplo excelente del patrón Mediator es un sistema de tráfico de una estación de tren. Dos trenes nunca se comunican entre sí para conocer la disponibilidad de una plataforma. El stationManager
actúa como mediador y pone la plataforma a disponibilidad únicamente de uno de los trenes entrantes mientras que pone al resto en cola. Un tren saliente informa a la estación, que permite entrar al siguiente tren en cola.
train.go: Componente
package main
type Train interface {
arrive()
depart()
permitArrival()
}
passengerTrain.go: Componente concreto
package main
import "fmt"
type PassengerTrain struct {
mediator Mediator
}
func (g *PassengerTrain) arrive() {
if !g.mediator.canArrive(g) {
fmt.Println("PassengerTrain: Arrival blocked, waiting")
return
}
fmt.Println("PassengerTrain: Arrived")
}
func (g *PassengerTrain) depart() {
fmt.Println("PassengerTrain: Leaving")
g.mediator.notifyAboutDeparture()
}
func (g *PassengerTrain) permitArrival() {
fmt.Println("PassengerTrain: Arrival permitted, arriving")
g.arrive()
}
freightTrain.go: Componente concreto
package main
import "fmt"
type FreightTrain struct {
mediator Mediator
}
func (g *FreightTrain) arrive() {
if !g.mediator.canArrive(g) {
fmt.Println("FreightTrain: Arrival blocked, waiting")
return
}
fmt.Println("FreightTrain: Arrived")
}
func (g *FreightTrain) depart() {
fmt.Println("FreightTrain: Leaving")
g.mediator.notifyAboutDeparture()
}
func (g *FreightTrain) permitArrival() {
fmt.Println("FreightTrain: Arrival permitted")
g.arrive()
}
mediator.go: Interfaz mediadora
package main
type Mediator interface {
canArrive(Train) bool
notifyAboutDeparture()
}
stationManager.go: Mediador concreto
package main
type StationManager struct {
isPlatformFree bool
trainQueue []Train
}
func newStationManger() *StationManager {
return &StationManager{
isPlatformFree: true,
}
}
func (s *StationManager) canArrive(t Train) bool {
if s.isPlatformFree {
s.isPlatformFree = false
return true
}
s.trainQueue = append(s.trainQueue, t)
return false
}
func (s *StationManager) notifyAboutDeparture() {
if !s.isPlatformFree {
s.isPlatformFree = true
}
if len(s.trainQueue) > 0 {
firstTrainInQueue := s.trainQueue[0]
s.trainQueue = s.trainQueue[1:]
firstTrainInQueue.permitArrival()
}
}
main.go: Código cliente
package main
func main() {
stationManager := newStationManger()
passengerTrain := &PassengerTrain{
mediator: stationManager,
}
freightTrain := &FreightTrain{
mediator: stationManager,
}
passengerTrain.arrive()
freightTrain.arrive()
passengerTrain.depart()
}
output.txt: Resultado de la ejecución
PassengerTrain: Arrived
FreightTrain: Arrival blocked, waiting
PassengerTrain: Leaving
FreightTrain: Arrival permitted
FreightTrain: Arrived