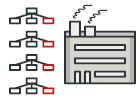
Abstract Factory を Java で
Abstract Factory は、 生成に関するデザインパターンのひとつで、 具象クラスを指定することなく、 プロダクト (訳注: 本パターンでは、 生成されるモノのことを一般にプロダクトと呼びます) のファミリー全部を生成することを可能とします。
Abstract Factory は、 個々のプロダクト全部を作成するためのインターフェースを定義しますが、 実際のプロダクト作成の作業は、 具象クラスに委ねられます。 ファクトリーの型 (クラス) それぞれは、 特定のプロダクトの異種に対応します。
クライアント・コードは、 コンストラクター呼び出し (new
演算子) で直接プロダクトを作成する代わりにファクトリー・オブジェクトの作成メソッドを呼び出します。 ファクトリーはプロダクトの特定の異種に対応しているため、 すべてのプロダクトには互換性があります。
クライアント・コードは、 その抽象インターフェイスを通じてのみファクトリーやプロダクトとやりとりします。 このため、 クライアント・コードはファクトリー・オブジェクトによって作成された任意のプロダクトの異種と動作します。 プログラマーがやるべきことは、 新しい具象ファクトリー・クラスを作成し、 それをクライアント・コードに渡すことです。
もし各種ファクトリー系のパターンやコンセプトの違いで迷った場合は、 ファクトリーの比較 をご覧ください。
複雑度:
人気度:
使用例: Abstract Factory パターンは、 Java コードではよく見かけます。 多くのフレームワークやライブラリーが、 その標準コンポーネントを拡張したりカスタマイズするためにこのパターンを使います。
Java のコア・ライブラリーでの使用例です:
見つけ方: このパターンは、 ファクトリー・オブジェクトを返すメソッドに注目すれば、 簡単に見つけられます。 そしてファクトリーを使ってサブコンポーネントが作成されます。
クロス・プラットフォーム GUI コンポーネントのファミリーとその作成方法
この例では、 ボタンとチェックボックスがプロダクトということになります。 これには macOS 用と Windows 用の 2 種類があります。
抽象ファクトリーは、 ボタンとチェックボックスを作成するためのインターフェースを定義します。 二つの具象ファクトリーがあり、 それぞれ同一種類のプロダクト二つを返します。
クライアント・コードは、 抽象インターフェースを使用してファクトリーやプロダクトと連携します。 このため、 同じクライアント・コードを使用して、 ファクトリー・オブジェクトの種類に応じて多種のプロダクトを扱うことができます。
buttons: 最初のプロダクトの階層
buttons/Button.java
package refactoring_guru.abstract_factory.example.buttons;
/**
* Abstract Factory assumes that you have several families of products,
* structured into separate class hierarchies (Button/Checkbox). All products of
* the same family have the common interface.
*
* This is the common interface for buttons family.
*/
public interface Button {
void paint();
}
buttons/MacOSButton.java
package refactoring_guru.abstract_factory.example.buttons;
/**
* All products families have the same varieties (MacOS/Windows).
*
* This is a MacOS variant of a button.
*/
public class MacOSButton implements Button {
@Override
public void paint() {
System.out.println("You have created MacOSButton.");
}
}
buttons/WindowsButton.java
package refactoring_guru.abstract_factory.example.buttons;
/**
* All products families have the same varieties (MacOS/Windows).
*
* This is another variant of a button.
*/
public class WindowsButton implements Button {
@Override
public void paint() {
System.out.println("You have created WindowsButton.");
}
}
checkboxes: 2 番目のプロダクトの階層
checkboxes/Checkbox.java
package refactoring_guru.abstract_factory.example.checkboxes;
/**
* Checkboxes is the second product family. It has the same variants as buttons.
*/
public interface Checkbox {
void paint();
}
checkboxes/MacOSCheckbox.java
package refactoring_guru.abstract_factory.example.checkboxes;
/**
* All products families have the same varieties (MacOS/Windows).
*
* This is a variant of a checkbox.
*/
public class MacOSCheckbox implements Checkbox {
@Override
public void paint() {
System.out.println("You have created MacOSCheckbox.");
}
}
checkboxes/WindowsCheckbox.java
package refactoring_guru.abstract_factory.example.checkboxes;
/**
* All products families have the same varieties (MacOS/Windows).
*
* This is another variant of a checkbox.
*/
public class WindowsCheckbox implements Checkbox {
@Override
public void paint() {
System.out.println("You have created WindowsCheckbox.");
}
}
factories
factories/GUIFactory.java: 抽象ファクトリー
package refactoring_guru.abstract_factory.example.factories;
import refactoring_guru.abstract_factory.example.buttons.Button;
import refactoring_guru.abstract_factory.example.checkboxes.Checkbox;
/**
* Abstract factory knows about all (abstract) product types.
*/
public interface GUIFactory {
Button createButton();
Checkbox createCheckbox();
}
factories/MacOSFactory.java: 具象ファクトリー (macOS)
package refactoring_guru.abstract_factory.example.factories;
import refactoring_guru.abstract_factory.example.buttons.Button;
import refactoring_guru.abstract_factory.example.buttons.MacOSButton;
import refactoring_guru.abstract_factory.example.checkboxes.Checkbox;
import refactoring_guru.abstract_factory.example.checkboxes.MacOSCheckbox;
/**
* Each concrete factory extends basic factory and responsible for creating
* products of a single variety.
*/
public class MacOSFactory implements GUIFactory {
@Override
public Button createButton() {
return new MacOSButton();
}
@Override
public Checkbox createCheckbox() {
return new MacOSCheckbox();
}
}
factories/WindowsFactory.java: 具象ファクトリー (Windows)
package refactoring_guru.abstract_factory.example.factories;
import refactoring_guru.abstract_factory.example.buttons.Button;
import refactoring_guru.abstract_factory.example.buttons.WindowsButton;
import refactoring_guru.abstract_factory.example.checkboxes.Checkbox;
import refactoring_guru.abstract_factory.example.checkboxes.WindowsCheckbox;
/**
* Each concrete factory extends basic factory and responsible for creating
* products of a single variety.
*/
public class WindowsFactory implements GUIFactory {
@Override
public Button createButton() {
return new WindowsButton();
}
@Override
public Checkbox createCheckbox() {
return new WindowsCheckbox();
}
}
app
app/Application.java: クライアント・コード
package refactoring_guru.abstract_factory.example.app;
import refactoring_guru.abstract_factory.example.buttons.Button;
import refactoring_guru.abstract_factory.example.checkboxes.Checkbox;
import refactoring_guru.abstract_factory.example.factories.GUIFactory;
/**
* Factory users don't care which concrete factory they use since they work with
* factories and products through abstract interfaces.
*/
public class Application {
private Button button;
private Checkbox checkbox;
public Application(GUIFactory factory) {
button = factory.createButton();
checkbox = factory.createCheckbox();
}
public void paint() {
button.paint();
checkbox.paint();
}
}
Demo.java: アプリの構成
package refactoring_guru.abstract_factory.example;
import refactoring_guru.abstract_factory.example.app.Application;
import refactoring_guru.abstract_factory.example.factories.GUIFactory;
import refactoring_guru.abstract_factory.example.factories.MacOSFactory;
import refactoring_guru.abstract_factory.example.factories.WindowsFactory;
/**
* Demo class. Everything comes together here.
*/
public class Demo {
/**
* Application picks the factory type and creates it in run time (usually at
* initialization stage), depending on the configuration or environment
* variables.
*/
private static Application configureApplication() {
Application app;
GUIFactory factory;
String osName = System.getProperty("os.name").toLowerCase();
if (osName.contains("mac")) {
factory = new MacOSFactory();
} else {
factory = new WindowsFactory();
}
app = new Application(factory);
return app;
}
public static void main(String[] args) {
Application app = configureApplication();
app.paint();
}
}
OutputDemo.txt: 実行結果
You create WindowsButton.
You created WindowsCheckbox.