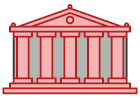
Facade を Java で
Facade は、 構造に関するデザインパターンの一つで、 複雑なクラスのシステム、 ライブラリー、 またはフレームワークに対して単純な (しかし限定された) インターフェースを提供します。
Facade は、 アプリケーションの全体としての複雑さを軽減しますが、 それと同時に望ましくない依存性を一箇所に集めるのにも役立ちます。
複雑度:
人気度:
使用例: Facade パターンは、 Java のアプリでよく見かけます。 複雑なライブラリーや API を相手にする時、 特に役に立ちます。
Java のコラ・ライブラリー内での Facade の使用例:
-
javax.faces.context.FacesContext
は、 実は内部でLifeCycle
、ViewHandler
、NavigationHandler
クラスを使用していますが、 ほとんどのクライアントはそれに気づいていません。 -
javax.faces.context.ExternalContext
は内部で、ServletContext
、HttpSession
、HttpServletRequest
、HttpServletResponse
他を使用します。
見つけ方: 単純なインターフェースのクラスがほとんどの作業を他のクラスに委任していたら、 Facade パターンの使用が識別できます。 通常、 ファサードは、 それが使うオブジェクトのライフサイクルを完全に管理します。
複雑なビデオ変換ライブラリー用の単純なインターフェース
この例では、 Facade パターンを使い、 複雑なビデオ変換フレームワークとのやりとりを簡素化します。
Facade を使用した結果のクラスは、 フレームワークの適切なクラスを構成し、 正しい形式で結果を取得するのに必要な複雑な処理すべてを行うメソッド一つからできています。
some_complex_media_library: 複雑なビデオ変換ライブラリー
some_complex_media_library/VideoFile.java
package refactoring_guru.facade.example.some_complex_media_library;
public class VideoFile {
private String name;
private String codecType;
public VideoFile(String name) {
this.name = name;
this.codecType = name.substring(name.indexOf(".") + 1);
}
public String getCodecType() {
return codecType;
}
public String getName() {
return name;
}
}
some_complex_media_library/Codec.java
package refactoring_guru.facade.example.some_complex_media_library;
public interface Codec {
}
some_complex_media_library/MPEG4CompressionCodec.java
package refactoring_guru.facade.example.some_complex_media_library;
public class MPEG4CompressionCodec implements Codec {
public String type = "mp4";
}
some_complex_media_library/OggCompressionCodec.java
package refactoring_guru.facade.example.some_complex_media_library;
public class OggCompressionCodec implements Codec {
public String type = "ogg";
}
some_complex_media_library/CodecFactory.java
package refactoring_guru.facade.example.some_complex_media_library;
public class CodecFactory {
public static Codec extract(VideoFile file) {
String type = file.getCodecType();
if (type.equals("mp4")) {
System.out.println("CodecFactory: extracting mpeg audio...");
return new MPEG4CompressionCodec();
}
else {
System.out.println("CodecFactory: extracting ogg audio...");
return new OggCompressionCodec();
}
}
}
some_complex_media_library/BitrateReader.java
package refactoring_guru.facade.example.some_complex_media_library;
public class BitrateReader {
public static VideoFile read(VideoFile file, Codec codec) {
System.out.println("BitrateReader: reading file...");
return file;
}
public static VideoFile convert(VideoFile buffer, Codec codec) {
System.out.println("BitrateReader: writing file...");
return buffer;
}
}
some_complex_media_library/AudioMixer.java
package refactoring_guru.facade.example.some_complex_media_library;
import java.io.File;
public class AudioMixer {
public File fix(VideoFile result){
System.out.println("AudioMixer: fixing audio...");
return new File("tmp");
}
}
facade
facade/VideoConversionFacade.java: Facade で、 ビデオ変換の単純なインターフェースを作成
package refactoring_guru.facade.example.facade;
import refactoring_guru.facade.example.some_complex_media_library.*;
import java.io.File;
public class VideoConversionFacade {
public File convertVideo(String fileName, String format) {
System.out.println("VideoConversionFacade: conversion started.");
VideoFile file = new VideoFile(fileName);
Codec sourceCodec = CodecFactory.extract(file);
Codec destinationCodec;
if (format.equals("mp4")) {
destinationCodec = new MPEG4CompressionCodec();
} else {
destinationCodec = new OggCompressionCodec();
}
VideoFile buffer = BitrateReader.read(file, sourceCodec);
VideoFile intermediateResult = BitrateReader.convert(buffer, destinationCodec);
File result = (new AudioMixer()).fix(intermediateResult);
System.out.println("VideoConversionFacade: conversion completed.");
return result;
}
}
Demo.java: クライアント・コード
package refactoring_guru.facade.example;
import refactoring_guru.facade.example.facade.VideoConversionFacade;
import java.io.File;
public class Demo {
public static void main(String[] args) {
VideoConversionFacade converter = new VideoConversionFacade();
File mp4Video = converter.convertVideo("youtubevideo.ogg", "mp4");
// ...
}
}
OutputDemo.txt: 実行結果
VideoConversionFacade: conversion started.
CodecFactory: extracting ogg audio...
BitrateReader: reading file...
BitrateReader: writing file...
AudioMixer: fixing audio...
VideoConversionFacade: conversion completed.