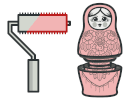
Decorator を Java で
Decorator は、 構造に関するパターンのひとつで、 オブジェクトをデコレーターと呼ばれる特別なラッパー・オブジェクト内に配置することにより、 新しい振る舞いを動的に追加できます。
対象のオブジェクトとデコレーターは同じインターフェースに従うため、 デコレーターを使うと、 オブジェクトを何重にも包み込むことができます。 その結果として生成されるオブジェクトは、 全部のラッパーの振る舞いを集積した振る舞いをします。
複雑度:
人気度:
使用例: Decorator は、 Java コードではかなり標準的で、 特にストリームに関連するコードではよく使われます。
Java のコア・ライブラリーでの Decorator の使用例です:
-
java.io.InputStream
、OutputStream
、Reader
、Writer
には、 自身と同じ型のオブジェクトを取るコンストラクターがあります。 -
java.util.Collections
、checkedXXX()
メソッド (以下同)、synchronizedXXX()
、unmodifiableXXX()
。 -
javax.servlet.http.HttpServletRequestWrapper
とHttpServletResponseWrapper
見つけ方: Decorator は、 そのクラスと同じクラスまたはインターフェースのオブジェクトをパラメーターとして取る生成メソッドかコンストラクターの存在により識別できます。
暗号化デコレーターと圧縮デコレーター
この例では、 コードを変更せずにオブジェクトの動作を変える方法を紹介します。
最初は、 ビジネス・ロジックのクラスは平文のテキストのデータの読み書きしかできませんでした。 次に、 ラップされたオブジェクトが標準操作を実行した後に新しい動作を追加するため、 小さなラッパー・クラスをいくつか作成しました。
最初のラッパーはデータの暗号化と復号化を行い、 二つ目のラッパーはデータの圧縮と解凍を行います。
デコレーターを別のデコレーターで包み込み、 組み合わせることさえできます。
decorators
decorators/DataSource.java: 読み書きの操作を定義する共通データ・インターフェース
package refactoring_guru.decorator.example.decorators;
public interface DataSource {
void writeData(String data);
String readData();
}
decorators/FileDataSource.java: 単純なデータの読み取り・書き取りクラス
package refactoring_guru.decorator.example.decorators;
import java.io.*;
public class FileDataSource implements DataSource {
private String name;
public FileDataSource(String name) {
this.name = name;
}
@Override
public void writeData(String data) {
File file = new File(name);
try (OutputStream fos = new FileOutputStream(file)) {
fos.write(data.getBytes(), 0, data.length());
} catch (IOException ex) {
System.out.println(ex.getMessage());
}
}
@Override
public String readData() {
char[] buffer = null;
File file = new File(name);
try (FileReader reader = new FileReader(file)) {
buffer = new char[(int) file.length()];
reader.read(buffer);
} catch (IOException ex) {
System.out.println(ex.getMessage());
}
return new String(buffer);
}
}
decorators/DataSourceDecorator.java: 抽象基底デコレーター
package refactoring_guru.decorator.example.decorators;
public abstract class DataSourceDecorator implements DataSource {
private DataSource wrappee;
DataSourceDecorator(DataSource source) {
this.wrappee = source;
}
@Override
public void writeData(String data) {
wrappee.writeData(data);
}
@Override
public String readData() {
return wrappee.readData();
}
}
decorators/EncryptionDecorator.java: 暗号化デコレーター
package refactoring_guru.decorator.example.decorators;
import java.util.Base64;
public class EncryptionDecorator extends DataSourceDecorator {
public EncryptionDecorator(DataSource source) {
super(source);
}
@Override
public void writeData(String data) {
super.writeData(encode(data));
}
@Override
public String readData() {
return decode(super.readData());
}
private String encode(String data) {
byte[] result = data.getBytes();
for (int i = 0; i < result.length; i++) {
result[i] += (byte) 1;
}
return Base64.getEncoder().encodeToString(result);
}
private String decode(String data) {
byte[] result = Base64.getDecoder().decode(data);
for (int i = 0; i < result.length; i++) {
result[i] -= (byte) 1;
}
return new String(result);
}
}
decorators/CompressionDecorator.java: 圧縮デコレーター
package refactoring_guru.decorator.example.decorators;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.util.Base64;
import java.util.zip.Deflater;
import java.util.zip.DeflaterOutputStream;
import java.util.zip.InflaterInputStream;
public class CompressionDecorator extends DataSourceDecorator {
private int compLevel = 6;
public CompressionDecorator(DataSource source) {
super(source);
}
public int getCompressionLevel() {
return compLevel;
}
public void setCompressionLevel(int value) {
compLevel = value;
}
@Override
public void writeData(String data) {
super.writeData(compress(data));
}
@Override
public String readData() {
return decompress(super.readData());
}
private String compress(String stringData) {
byte[] data = stringData.getBytes();
try {
ByteArrayOutputStream bout = new ByteArrayOutputStream(512);
DeflaterOutputStream dos = new DeflaterOutputStream(bout, new Deflater(compLevel));
dos.write(data);
dos.close();
bout.close();
return Base64.getEncoder().encodeToString(bout.toByteArray());
} catch (IOException ex) {
return null;
}
}
private String decompress(String stringData) {
byte[] data = Base64.getDecoder().decode(stringData);
try {
InputStream in = new ByteArrayInputStream(data);
InflaterInputStream iin = new InflaterInputStream(in);
ByteArrayOutputStream bout = new ByteArrayOutputStream(512);
int b;
while ((b = iin.read()) != -1) {
bout.write(b);
}
in.close();
iin.close();
bout.close();
return new String(bout.toByteArray());
} catch (IOException ex) {
return null;
}
}
}
Demo.java: クライアント・コード
package refactoring_guru.decorator.example;
import refactoring_guru.decorator.example.decorators.*;
public class Demo {
public static void main(String[] args) {
String salaryRecords = "Name,Salary\nJohn Smith,100000\nSteven Jobs,912000";
DataSourceDecorator encoded = new CompressionDecorator(
new EncryptionDecorator(
new FileDataSource("out/OutputDemo.txt")));
encoded.writeData(salaryRecords);
DataSource plain = new FileDataSource("out/OutputDemo.txt");
System.out.println("- Input ----------------");
System.out.println(salaryRecords);
System.out.println("- Encoded --------------");
System.out.println(plain.readData());
System.out.println("- Decoded --------------");
System.out.println(encoded.readData());
}
}
OutputDemo.txt: 実行結果
- Input ----------------
Name,Salary
John Smith,100000
Steven Jobs,912000
- Encoded --------------
Zkt7e1Q5eU8yUm1Qe0ZsdHJ2VXp6dDBKVnhrUHtUe0sxRUYxQkJIdjVLTVZ0dVI5Q2IwOXFISmVUMU5rcENCQmdxRlByaD4+
- Decoded --------------
Name,Salary
John Smith,100000
Steven Jobs,912000