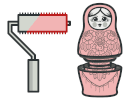
Decorator を Go で
Decorator は、 構造に関するパターンのひとつで、 オブジェクトをデコレーターと呼ばれる特別なラッパー・オブジェクト内に配置することにより、 新しい振る舞いを動的に追加できます。
対象のオブジェクトとデコレーターは同じインターフェースに従うため、 デコレーターを使うと、 オブジェクトを何重にも包み込むことができます。 その結果として生成されるオブジェクトは、 全部のラッパーの振る舞いを集積した振る舞いをします。
概念的な例
pizza.go: コンポーネントのインターフェース
package main
type IPizza interface {
getPrice() int
}
veggieMania.go: 具象コンポーネント
package main
type VeggieMania struct {
}
func (p *VeggieMania) getPrice() int {
return 15
}
tomatoTopping.go: 具象デコレーター
package main
type TomatoTopping struct {
pizza IPizza
}
func (c *TomatoTopping) getPrice() int {
pizzaPrice := c.pizza.getPrice()
return pizzaPrice + 7
}
cheeseTopping.go: 具象デコレーター
package main
type CheeseTopping struct {
pizza IPizza
}
func (c *CheeseTopping) getPrice() int {
pizzaPrice := c.pizza.getPrice()
return pizzaPrice + 10
}
main.go: クライアント・コード
package main
import "fmt"
func main() {
pizza := &VeggieMania{}
//Add cheese topping
pizzaWithCheese := &CheeseTopping{
pizza: pizza,
}
//Add tomato topping
pizzaWithCheeseAndTomato := &TomatoTopping{
pizza: pizzaWithCheese,
}
fmt.Printf("Price of veggeMania with tomato and cheese topping is %d\n", pizzaWithCheeseAndTomato.getPrice())
}
output.txt: 実行結果
Price of veggeMania with tomato and cheese topping is 32