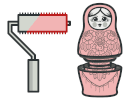
Go로 작성된 데코레이터
데코레이터는 구조 패턴이며 새로운 행동들을 특수 래퍼 객체들 내에 넣어서 이러한 행동들을 객체들에 동적으로 추가할 수 있도록 합니다.
데코레이터를 사용하여 객체들을 제한 없이 래핑할 수 있습니다. 왜냐하면 대상 객체들과 데코레이터들은 같은 인터페이스를 따르기 때문입니다. 결과 객체는 모든 래퍼의 스태킹된 행동을 가질 것입니다.
개념적인 예시
pizza.go: 컴포넌트 인터페이스
package main
type IPizza interface {
getPrice() int
}
veggieMania.go: 구상 컴포넌트
package main
type VeggieMania struct {
}
func (p *VeggieMania) getPrice() int {
return 15
}
tomatoTopping.go: 구상 데코레이터
package main
type TomatoTopping struct {
pizza IPizza
}
func (c *TomatoTopping) getPrice() int {
pizzaPrice := c.pizza.getPrice()
return pizzaPrice + 7
}
cheeseTopping.go: 구상 데코레이터
package main
type CheeseTopping struct {
pizza IPizza
}
func (c *CheeseTopping) getPrice() int {
pizzaPrice := c.pizza.getPrice()
return pizzaPrice + 10
}
main.go: 클라이언트 코드
package main
import "fmt"
func main() {
pizza := &VeggieMania{}
//Add cheese topping
pizzaWithCheese := &CheeseTopping{
pizza: pizza,
}
//Add tomato topping
pizzaWithCheeseAndTomato := &TomatoTopping{
pizza: pizzaWithCheese,
}
fmt.Printf("Price of veggeMania with tomato and cheese topping is %d\n", pizzaWithCheeseAndTomato.getPrice())
}
output.txt: 실행 결과
Price of veggeMania with tomato and cheese topping is 32