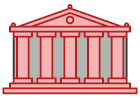
Go로 작성된 퍼사드
퍼사드는 구조 디자인 패턴이며 간단한 (그러나 제한된 인터페이스를 클래스, 라이브러리 또는 프레임워크로 구성된 복잡한 시스템에 제공합니다.
퍼사드는 앱의 전반적인 복잡성을 줄이는 동시에 원치 않는 의존성들을 한 곳으로 옮기는 것을 돕습니다.
개념적인 예시
신용 카드를 사용하여 피자를 주문할 때 시스템 내부에서 일어나는 프로세스의 복잡성을 과소평가하기 쉽습니다. 이 프로세스 내에 작동하는 수십 개의 하위 시스템이 있으며 다음은 그중 일부입니다:
- 계정 확인
- 보안 PIN 확인
- 대변/차변 잔액
- 원장 입력
- 알림 보내기
이와 같은 복잡한 시스템을 탐색하기 쉽지 않으며 또 잘못된 작업을 수행하면 코드가 깨지기 쉽습니다. 이것이 클라이언트가 간단한 인터페이스를 사용하여 수십 개의 구성 요소와 작업할 수 있도록 하는 퍼사드 패턴의 개념이 있는 이유입니다. 클라이언트는 카드 정보, 보안 핀, 지불 금액 및 작업 유형만 입력하면 됩니다. 퍼사드는 클라이언트를 내부 복잡성에 노출하지 않고 다양한 구성 요소와의 추가 통신을 지시합니다.
walletFacade.go: 퍼사드
package main
import "fmt"
type WalletFacade struct {
account *Account
wallet *Wallet
securityCode *SecurityCode
notification *Notification
ledger *Ledger
}
func newWalletFacade(accountID string, code int) *WalletFacade {
fmt.Println("Starting create account")
walletFacacde := &WalletFacade{
account: newAccount(accountID),
securityCode: newSecurityCode(code),
wallet: newWallet(),
notification: &Notification{},
ledger: &Ledger{},
}
fmt.Println("Account created")
return walletFacacde
}
func (w *WalletFacade) addMoneyToWallet(accountID string, securityCode int, amount int) error {
fmt.Println("Starting add money to wallet")
err := w.account.checkAccount(accountID)
if err != nil {
return err
}
err = w.securityCode.checkCode(securityCode)
if err != nil {
return err
}
w.wallet.creditBalance(amount)
w.notification.sendWalletCreditNotification()
w.ledger.makeEntry(accountID, "credit", amount)
return nil
}
func (w *WalletFacade) deductMoneyFromWallet(accountID string, securityCode int, amount int) error {
fmt.Println("Starting debit money from wallet")
err := w.account.checkAccount(accountID)
if err != nil {
return err
}
err = w.securityCode.checkCode(securityCode)
if err != nil {
return err
}
err = w.wallet.debitBalance(amount)
if err != nil {
return err
}
w.notification.sendWalletDebitNotification()
w.ledger.makeEntry(accountID, "debit", amount)
return nil
}
account.go: 복잡한 하위 시스템 부품들
package main
import "fmt"
type Account struct {
name string
}
func newAccount(accountName string) *Account {
return &Account{
name: accountName,
}
}
func (a *Account) checkAccount(accountName string) error {
if a.name != accountName {
return fmt.Errorf("Account Name is incorrect")
}
fmt.Println("Account Verified")
return nil
}
securityCode.go: 복잡한 하위 시스템 부품들
package main
import "fmt"
type SecurityCode struct {
code int
}
func newSecurityCode(code int) *SecurityCode {
return &SecurityCode{
code: code,
}
}
func (s *SecurityCode) checkCode(incomingCode int) error {
if s.code != incomingCode {
return fmt.Errorf("Security Code is incorrect")
}
fmt.Println("SecurityCode Verified")
return nil
}
wallet.go: 복잡한 하위 시스템 부품들
package main
import "fmt"
type Wallet struct {
balance int
}
func newWallet() *Wallet {
return &Wallet{
balance: 0,
}
}
func (w *Wallet) creditBalance(amount int) {
w.balance += amount
fmt.Println("Wallet balance added successfully")
return
}
func (w *Wallet) debitBalance(amount int) error {
if w.balance < amount {
return fmt.Errorf("Balance is not sufficient")
}
fmt.Println("Wallet balance is Sufficient")
w.balance = w.balance - amount
return nil
}
ledger.go: 복잡한 하위 시스템 부품들
package main
import "fmt"
type Ledger struct {
}
func (s *Ledger) makeEntry(accountID, txnType string, amount int) {
fmt.Printf("Make ledger entry for accountId %s with txnType %s for amount %d\n", accountID, txnType, amount)
return
}
notification.go: 복잡한 하위 시스템 부품들
package main
import "fmt"
type Notification struct {
}
func (n *Notification) sendWalletCreditNotification() {
fmt.Println("Sending wallet credit notification")
}
func (n *Notification) sendWalletDebitNotification() {
fmt.Println("Sending wallet debit notification")
}
main.go: 클라이언트 코드
package main
import (
"fmt"
"log"
)
func main() {
fmt.Println()
walletFacade := newWalletFacade("abc", 1234)
fmt.Println()
err := walletFacade.addMoneyToWallet("abc", 1234, 10)
if err != nil {
log.Fatalf("Error: %s\n", err.Error())
}
fmt.Println()
err = walletFacade.deductMoneyFromWallet("abc", 1234, 5)
if err != nil {
log.Fatalf("Error: %s\n", err.Error())
}
}
output.txt: 실행 결과
Starting create account
Account created
Starting add money to wallet
Account Verified
SecurityCode Verified
Wallet balance added successfully
Sending wallet credit notification
Make ledger entry for accountId abc with txnType credit for amount 10
Starting debit money from wallet
Account Verified
SecurityCode Verified
Wallet balance is Sufficient
Sending wallet debit notification
Make ledger entry for accountId abc with txnType debit for amount 5