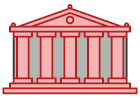
파이썬으로 작성된 퍼사드
퍼사드는 구조 디자인 패턴이며 간단한 (그러나 제한된 인터페이스를 클래스, 라이브러리 또는 프레임워크로 구성된 복잡한 시스템에 제공합니다.
퍼사드는 앱의 전반적인 복잡성을 줄이는 동시에 원치 않는 의존성들을 한 곳으로 옮기는 것을 돕습니다.
복잡도:
인기도:
사용 사례들: 퍼사드 패턴은 일반적으로 파이썬 코드로 작성된 앱에서 사용되며 또 복잡한 라이브러리 및 API와 작업할 때 특히 편리합니다.
식별: 퍼사드는 인터페이스가 간단하나 대부분의 작업을 다른 클래스들에 위임하는 클래스의 존재여부로 식별할 수 있으며 일반적으로 퍼사드들은 사용하는 객체들의 전체 수명 주기를 관리합니다.
개념적인 예시
이 예시는 퍼사드 패턴의 구조를 보여주고 다음 질문에 중점을 둡니다:
- 패턴은 어떤 클래스들로 구성되어 있나요?
- 이 클래스들은 어떤 역할을 하나요?
- 패턴의 요소들은 어떻게 서로 연관되어 있나요?
main.py: 개념적인 예시
from __future__ import annotations
class Facade:
"""
The Facade class provides a simple interface to the complex logic of one or
several subsystems. The Facade delegates the client requests to the
appropriate objects within the subsystem. The Facade is also responsible for
managing their lifecycle. All of this shields the client from the undesired
complexity of the subsystem.
"""
def __init__(self, subsystem1: Subsystem1, subsystem2: Subsystem2) -> None:
"""
Depending on your application's needs, you can provide the Facade with
existing subsystem objects or force the Facade to create them on its
own.
"""
self._subsystem1 = subsystem1 or Subsystem1()
self._subsystem2 = subsystem2 or Subsystem2()
def operation(self) -> str:
"""
The Facade's methods are convenient shortcuts to the sophisticated
functionality of the subsystems. However, clients get only to a fraction
of a subsystem's capabilities.
"""
results = []
results.append("Facade initializes subsystems:")
results.append(self._subsystem1.operation1())
results.append(self._subsystem2.operation1())
results.append("Facade orders subsystems to perform the action:")
results.append(self._subsystem1.operation_n())
results.append(self._subsystem2.operation_z())
return "\n".join(results)
class Subsystem1:
"""
The Subsystem can accept requests either from the facade or client directly.
In any case, to the Subsystem, the Facade is yet another client, and it's
not a part of the Subsystem.
"""
def operation1(self) -> str:
return "Subsystem1: Ready!"
# ...
def operation_n(self) -> str:
return "Subsystem1: Go!"
class Subsystem2:
"""
Some facades can work with multiple subsystems at the same time.
"""
def operation1(self) -> str:
return "Subsystem2: Get ready!"
# ...
def operation_z(self) -> str:
return "Subsystem2: Fire!"
def client_code(facade: Facade) -> None:
"""
The client code works with complex subsystems through a simple interface
provided by the Facade. When a facade manages the lifecycle of the
subsystem, the client might not even know about the existence of the
subsystem. This approach lets you keep the complexity under control.
"""
print(facade.operation(), end="")
if __name__ == "__main__":
# The client code may have some of the subsystem's objects already created.
# In this case, it might be worthwhile to initialize the Facade with these
# objects instead of letting the Facade create new instances.
subsystem1 = Subsystem1()
subsystem2 = Subsystem2()
facade = Facade(subsystem1, subsystem2)
client_code(facade)
Output.txt: 실행 결과
Facade initializes subsystems:
Subsystem1: Ready!
Subsystem2: Get ready!
Facade orders subsystems to perform the action:
Subsystem1: Go!
Subsystem2: Fire!