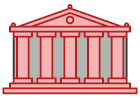
Facade in Python
Facade is a structural design pattern that provides a simplified (but limited) interface to a complex system of classes, library or framework.
While Facade decreases the overall complexity of the application, it also helps to move unwanted dependencies to one place.
Complexity:
Popularity:
Usage examples: The Facade pattern is commonly used in apps written in Python. It’s especially handy when working with complex libraries and APIs.
Identification: Facade can be recognized in a class that has a simple interface, but delegates most of the work to other classes. Usually, facades manage the full life cycle of objects they use.
Conceptual Example
This example illustrates the structure of the Facade design pattern. It focuses on answering these questions:
- What classes does it consist of?
- What roles do these classes play?
- In what way the elements of the pattern are related?
main.py: Conceptual example
from __future__ import annotations
class Facade:
"""
The Facade class provides a simple interface to the complex logic of one or
several subsystems. The Facade delegates the client requests to the
appropriate objects within the subsystem. The Facade is also responsible for
managing their lifecycle. All of this shields the client from the undesired
complexity of the subsystem.
"""
def __init__(self, subsystem1: Subsystem1, subsystem2: Subsystem2) -> None:
"""
Depending on your application's needs, you can provide the Facade with
existing subsystem objects or force the Facade to create them on its
own.
"""
self._subsystem1 = subsystem1 or Subsystem1()
self._subsystem2 = subsystem2 or Subsystem2()
def operation(self) -> str:
"""
The Facade's methods are convenient shortcuts to the sophisticated
functionality of the subsystems. However, clients get only to a fraction
of a subsystem's capabilities.
"""
results = []
results.append("Facade initializes subsystems:")
results.append(self._subsystem1.operation1())
results.append(self._subsystem2.operation1())
results.append("Facade orders subsystems to perform the action:")
results.append(self._subsystem1.operation_n())
results.append(self._subsystem2.operation_z())
return "\n".join(results)
class Subsystem1:
"""
The Subsystem can accept requests either from the facade or client directly.
In any case, to the Subsystem, the Facade is yet another client, and it's
not a part of the Subsystem.
"""
def operation1(self) -> str:
return "Subsystem1: Ready!"
# ...
def operation_n(self) -> str:
return "Subsystem1: Go!"
class Subsystem2:
"""
Some facades can work with multiple subsystems at the same time.
"""
def operation1(self) -> str:
return "Subsystem2: Get ready!"
# ...
def operation_z(self) -> str:
return "Subsystem2: Fire!"
def client_code(facade: Facade) -> None:
"""
The client code works with complex subsystems through a simple interface
provided by the Facade. When a facade manages the lifecycle of the
subsystem, the client might not even know about the existence of the
subsystem. This approach lets you keep the complexity under control.
"""
print(facade.operation(), end="")
if __name__ == "__main__":
# The client code may have some of the subsystem's objects already created.
# In this case, it might be worthwhile to initialize the Facade with these
# objects instead of letting the Facade create new instances.
subsystem1 = Subsystem1()
subsystem2 = Subsystem2()
facade = Facade(subsystem1, subsystem2)
client_code(facade)
Output.txt: Execution result
Facade initializes subsystems:
Subsystem1: Ready!
Subsystem2: Get ready!
Facade orders subsystems to perform the action:
Subsystem1: Go!
Subsystem2: Fire!