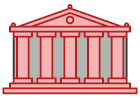
Facade in Ruby
Facade is a structural design pattern that provides a simplified (but limited) interface to a complex system of classes, library or framework.
While Facade decreases the overall complexity of the application, it also helps to move unwanted dependencies to one place.
Complexity:
Popularity:
Usage examples: The Facade pattern is commonly used in apps written in Ruby. It’s especially handy when working with complex libraries and APIs.
Identification: Facade can be recognized in a class that has a simple interface, but delegates most of the work to other classes. Usually, facades manage the full life cycle of objects they use.
Conceptual Example
This example illustrates the structure of the Facade design pattern. It focuses on answering these questions:
- What classes does it consist of?
- What roles do these classes play?
- In what way the elements of the pattern are related?
main.rb: Conceptual example
# The Facade class provides a simple interface to the complex logic of one or
# several subsystems. The Facade delegates the client requests to the
# appropriate objects within the subsystem. The Facade is also responsible for
# managing their lifecycle. All of this shields the client from the undesired
# complexity of the subsystem.
class Facade
# Depending on your application's needs, you can provide the Facade with
# existing subsystem objects or force the Facade to create them on its own.
def initialize(subsystem1, subsystem2)
@subsystem1 = subsystem1 || Subsystem1.new
@subsystem2 = subsystem2 || Subsystem2.new
end
# The Facade's methods are convenient shortcuts to the sophisticated
# functionality of the subsystems. However, clients get only to a fraction of
# a subsystem's capabilities.
def operation
results = []
results.append('Facade initializes subsystems:')
results.append(@subsystem1.operation1)
results.append(@subsystem2.operation1)
results.append('Facade orders subsystems to perform the action:')
results.append(@subsystem1.operation_n)
results.append(@subsystem2.operation_z)
results.join("\n")
end
end
# The Subsystem can accept requests either from the facade or client directly.
# In any case, to the Subsystem, the Facade is yet another client, and it's not
# a part of the Subsystem.
class Subsystem1
# @return [String]
def operation1
'Subsystem1: Ready!'
end
# ...
# @return [String]
def operation_n
'Subsystem1: Go!'
end
end
# Some facades can work with multiple subsystems at the same time.
class Subsystem2
# @return [String]
def operation1
'Subsystem2: Get ready!'
end
# ...
# @return [String]
def operation_z
'Subsystem2: Fire!'
end
end
# The client code works with complex subsystems through a simple interface
# provided by the Facade. When a facade manages the lifecycle of the subsystem,
# the client might not even know about the existence of the subsystem. This
# approach lets you keep the complexity under control.
def client_code(facade)
print facade.operation
end
# The client code may have some of the subsystem's objects already created. In
# this case, it might be worthwhile to initialize the Facade with these objects
# instead of letting the Facade create new instances.
subsystem1 = Subsystem1.new
subsystem2 = Subsystem2.new
facade = Facade.new(subsystem1, subsystem2)
client_code(facade)
output.txt: Execution result
Facade initializes subsystems:
Subsystem1: Ready!
Subsystem2: Get ready!
Facade orders subsystems to perform the action:
Subsystem1: Go!
Subsystem2: Fire!