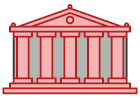
자바로 작성된 퍼사드
퍼사드는 구조 디자인 패턴이며 간단한 (그러나 제한된 인터페이스를 클래스, 라이브러리 또는 프레임워크로 구성된 복잡한 시스템에 제공합니다.
퍼사드는 앱의 전반적인 복잡성을 줄이는 동시에 원치 않는 의존성들을 한 곳으로 옮기는 것을 돕습니다.
복잡도:
인기도:
사용 사례들: 퍼사드 패턴은 일반적으로 자바로 작성된 앱에서 사용되며 또 복잡한 라이브러리 및 API와 작업할 때 특히 편리합니다.
다음은 코어 자바 라이브러리로부터 가져온 몇 가지 퍼사드 예시들입니다:
-
javax.faces.context.FacesContext
는 내부적으로LifeCycle
,ViewHandler
,NavigationHandler
클래스들을 사용하나, 대부분 클라이언트는 이러한 사실을 모릅니다. -
javax.faces.context.ExternalContext
는ServletContext
,HttpSession
,HttpServletRequest
,HttpServletResponse
등을 내부에서 사용합니다.
식별: 퍼사드는 인터페이스가 간단하나 대부분의 작업을 다른 클래스들에 위임하는 클래스의 존재여부로 식별할 수 있으며 일반적으로 퍼사드들은 사용하는 객체들의 전체 수명 주기를 관리합니다.
복잡한 비디오 변환 라이브러리를 위한 간단한 인터페이스
이 예시에서 퍼사드 패턴은 복잡한 비디오 변환 프레임워크와의 통신을 단순화합니다.
퍼사드는 하나의 메서드를 가진 단일 메서드를 제공하며 이 메서드는 프레임워크의 올바른 클래스들의 설정 그리고 결과를 가져오는 작업에 대한 모든 복잡성을 처리합니다.
some_complex_media_library: 복잡한 비디오 변환 라이브러리
some_complex_media_library/VideoFile.java
package refactoring_guru.facade.example.some_complex_media_library;
public class VideoFile {
private String name;
private String codecType;
public VideoFile(String name) {
this.name = name;
this.codecType = name.substring(name.indexOf(".") + 1);
}
public String getCodecType() {
return codecType;
}
public String getName() {
return name;
}
}
some_complex_media_library/Codec.java
package refactoring_guru.facade.example.some_complex_media_library;
public interface Codec {
}
some_complex_media_library/MPEG4CompressionCodec.java
package refactoring_guru.facade.example.some_complex_media_library;
public class MPEG4CompressionCodec implements Codec {
public String type = "mp4";
}
some_complex_media_library/OggCompressionCodec.java
package refactoring_guru.facade.example.some_complex_media_library;
public class OggCompressionCodec implements Codec {
public String type = "ogg";
}
some_complex_media_library/CodecFactory.java
package refactoring_guru.facade.example.some_complex_media_library;
public class CodecFactory {
public static Codec extract(VideoFile file) {
String type = file.getCodecType();
if (type.equals("mp4")) {
System.out.println("CodecFactory: extracting mpeg audio...");
return new MPEG4CompressionCodec();
}
else {
System.out.println("CodecFactory: extracting ogg audio...");
return new OggCompressionCodec();
}
}
}
some_complex_media_library/BitrateReader.java
package refactoring_guru.facade.example.some_complex_media_library;
public class BitrateReader {
public static VideoFile read(VideoFile file, Codec codec) {
System.out.println("BitrateReader: reading file...");
return file;
}
public static VideoFile convert(VideoFile buffer, Codec codec) {
System.out.println("BitrateReader: writing file...");
return buffer;
}
}
some_complex_media_library/AudioMixer.java
package refactoring_guru.facade.example.some_complex_media_library;
import java.io.File;
public class AudioMixer {
public File fix(VideoFile result){
System.out.println("AudioMixer: fixing audio...");
return new File("tmp");
}
}
facade
facade/VideoConversionFacade.java: 퍼사드는 비디오 변환의 간단한 인터페이스를 제공합니다
package refactoring_guru.facade.example.facade;
import refactoring_guru.facade.example.some_complex_media_library.*;
import java.io.File;
public class VideoConversionFacade {
public File convertVideo(String fileName, String format) {
System.out.println("VideoConversionFacade: conversion started.");
VideoFile file = new VideoFile(fileName);
Codec sourceCodec = CodecFactory.extract(file);
Codec destinationCodec;
if (format.equals("mp4")) {
destinationCodec = new MPEG4CompressionCodec();
} else {
destinationCodec = new OggCompressionCodec();
}
VideoFile buffer = BitrateReader.read(file, sourceCodec);
VideoFile intermediateResult = BitrateReader.convert(buffer, destinationCodec);
File result = (new AudioMixer()).fix(intermediateResult);
System.out.println("VideoConversionFacade: conversion completed.");
return result;
}
}
Demo.java: 클라이언트 코드
package refactoring_guru.facade.example;
import refactoring_guru.facade.example.facade.VideoConversionFacade;
import java.io.File;
public class Demo {
public static void main(String[] args) {
VideoConversionFacade converter = new VideoConversionFacade();
File mp4Video = converter.convertVideo("youtubevideo.ogg", "mp4");
// ...
}
}
OutputDemo.txt: 실행 결과
VideoConversionFacade: conversion started.
CodecFactory: extracting ogg audio...
BitrateReader: reading file...
BitrateReader: writing file...
AudioMixer: fixing audio...
VideoConversionFacade: conversion completed.