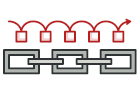
PHP로 작성된 책임 연쇄
책임 연쇄 패턴은 핸들러 중 하나가 요청을 처리할 때까지 핸들러들의 체인(사슬)을 따라 요청을 전달할 수 있게 해주는 행동 디자인 패턴입니다.
이 패턴은 발신자 클래스를 수신자들의 구상 클래스들에 연결하지 않고도 여러 객체가 요청을 처리할 수 있도록 합니다. 체인은 표준 핸들러 인터페이스를 따르는 모든 핸들러와 런타임 때 동적으로 구성될 수 있습니다.
복잡도:
인기도:
사용 예시들: 책임 연쇄 패턴은 다양한 PHP 프레임워크에서 매우 일반적입니다. PHP에서 이 패턴을 사용하는 가장 유명한 예 중 하나는 PSR -15에 설명된 HTTP 요청 미들웨어입니다.
식별: 패턴의 모든 객체는 공통 인터페이스를 따르며, 다른 객체들의 같은 메서드들을 간접적으로 호출하는 한 객체 그룹의 행동 메서드들이 있습니다.
개념적인 예시
이 예시는 책임 연쇄 패턴의 구조를 보여주고 다음 질문에 중점을 둡니다:
- 패턴은 어떤 클래스들로 구성되어 있나요?
- 이 클래스들은 어떤 역할을 하나요?
- 패턴의 요소들은 어떻게 서로 연관되어 있나요?
이 패턴의 구조를 배우면 실제 PHP 사용 사례를 기반으로 하는 다음 예시를 더욱 쉽게 이해할 수 있을 것입니다.
index.php: 개념적인 예시
<?php
namespace RefactoringGuru\ChainOfResponsibility\Conceptual;
/**
* The Handler interface declares a method for building the chain of handlers.
* It also declares a method for executing a request.
*/
interface Handler
{
public function setNext(Handler $handler): Handler;
public function handle(string $request): ?string;
}
/**
* The default chaining behavior can be implemented inside a base handler class.
*/
abstract class AbstractHandler implements Handler
{
/**
* @var Handler
*/
private $nextHandler;
public function setNext(Handler $handler): Handler
{
$this->nextHandler = $handler;
// Returning a handler from here will let us link handlers in a
// convenient way like this:
// $monkey->setNext($squirrel)->setNext($dog);
return $handler;
}
public function handle(string $request): ?string
{
if ($this->nextHandler) {
return $this->nextHandler->handle($request);
}
return null;
}
}
/**
* All Concrete Handlers either handle a request or pass it to the next handler
* in the chain.
*/
class MonkeyHandler extends AbstractHandler
{
public function handle(string $request): ?string
{
if ($request === "Banana") {
return "Monkey: I'll eat the " . $request . ".\n";
} else {
return parent::handle($request);
}
}
}
class SquirrelHandler extends AbstractHandler
{
public function handle(string $request): ?string
{
if ($request === "Nut") {
return "Squirrel: I'll eat the " . $request . ".\n";
} else {
return parent::handle($request);
}
}
}
class DogHandler extends AbstractHandler
{
public function handle(string $request): ?string
{
if ($request === "MeatBall") {
return "Dog: I'll eat the " . $request . ".\n";
} else {
return parent::handle($request);
}
}
}
/**
* The client code is usually suited to work with a single handler. In most
* cases, it is not even aware that the handler is part of a chain.
*/
function clientCode(Handler $handler)
{
foreach (["Nut", "Banana", "Cup of coffee"] as $food) {
echo "Client: Who wants a " . $food . "?\n";
$result = $handler->handle($food);
if ($result) {
echo " " . $result;
} else {
echo " " . $food . " was left untouched.\n";
}
}
}
/**
* The other part of the client code constructs the actual chain.
*/
$monkey = new MonkeyHandler();
$squirrel = new SquirrelHandler();
$dog = new DogHandler();
$monkey->setNext($squirrel)->setNext($dog);
/**
* The client should be able to send a request to any handler, not just the
* first one in the chain.
*/
echo "Chain: Monkey > Squirrel > Dog\n\n";
clientCode($monkey);
echo "\n";
echo "Subchain: Squirrel > Dog\n\n";
clientCode($squirrel);
Output.txt: 실행 결과
Chain: Monkey > Squirrel > Dog
Client: Who wants a Nut?
Squirrel: I'll eat the Nut.
Client: Who wants a Banana?
Monkey: I'll eat the Banana.
Client: Who wants a Cup of coffee?
Cup of coffee was left untouched.
Subchain: Squirrel > Dog
Client: Who wants a Nut?
Squirrel: I'll eat the Nut.
Client: Who wants a Banana?
Banana was left untouched.
Client: Who wants a Cup of coffee?
Cup of coffee was left untouched.
실제 사례 예시
PHP 세계에서 가장 널리 알려진 책임 연쇄(CoR) 패턴은 HTTP 요청 미들웨어에서 볼 수 있습니다. 이러한 미들웨어들은 가장 인기 있는 PHP 프레임워크에 의해 구현되며 PSR -15의 일부로 표준화되었습니다.
위는 다음과 같이 작동합니다: HTTP 요청은 앱에서 처리되기 위해 미들웨어 객체들의 스택을 통과해야 합니다. 앱에서 처리하려면 HTTP 요청이 미들웨어 개체 스택을 통과해야 합니다. 각 미들웨어는 요청의 추가 처리를 거부하거나 다음 미들웨어로 전달할 수 있습니다. 요청이 모든 미들웨어를 성공적으로 통과하면 앱의 기본 핸들러가 마침내 이를 처리할 수 있습니다.
이 접근 방식이 패턴의 원래 의도와 약간 반대라는 것을 눈치채셨을 수도 있습니다. 실제로 일반적인 구현에서 요청은 현재 핸들러가 해당 요청을 처리할 수 없는 경우에만 체인을 따라 전달됩니다. 미들웨어는 앱이 요청을 처리할 수 있다고 생각할 때 요청을 체인 아래로 더 전달합니다. 그럼에도 불구하고 미들웨어 객체들이 사슬식으로 연결되어 있으므로 이 개념은 여전히 책임 연쇄 패턴의 예시로 간주됩니다.
index.php: 실제 사례 예시
<?php
namespace RefactoringGuru\ChainOfResponsibility\RealWorld;
/**
* The classic CoR pattern declares a single role for objects that make up a
* chain, which is a Handler. In our example, let's differentiate between
* middleware and a final application's handler, which is executed when a
* request gets through all the middleware objects.
*
* The base Middleware class declares an interface for linking middleware
* objects into a chain.
*/
abstract class Middleware
{
/**
* @var Middleware
*/
private $next;
/**
* This method can be used to build a chain of middleware objects.
*/
public function linkWith(Middleware $next): Middleware
{
$this->next = $next;
return $next;
}
/**
* Subclasses must override this method to provide their own checks. A
* subclass can fall back to the parent implementation if it can't process a
* request.
*/
public function check(string $email, string $password): bool
{
if (!$this->next) {
return true;
}
return $this->next->check($email, $password);
}
}
/**
* This Concrete Middleware checks whether a user with given credentials exists.
*/
class UserExistsMiddleware extends Middleware
{
private $server;
public function __construct(Server $server)
{
$this->server = $server;
}
public function check(string $email, string $password): bool
{
if (!$this->server->hasEmail($email)) {
echo "UserExistsMiddleware: This email is not registered!\n";
return false;
}
if (!$this->server->isValidPassword($email, $password)) {
echo "UserExistsMiddleware: Wrong password!\n";
return false;
}
return parent::check($email, $password);
}
}
/**
* This Concrete Middleware checks whether a user associated with the request
* has sufficient permissions.
*/
class RoleCheckMiddleware extends Middleware
{
public function check(string $email, string $password): bool
{
if ($email === "admin@example.com") {
echo "RoleCheckMiddleware: Hello, admin!\n";
return true;
}
echo "RoleCheckMiddleware: Hello, user!\n";
return parent::check($email, $password);
}
}
/**
* This Concrete Middleware checks whether there are too many failed login
* requests.
*/
class ThrottlingMiddleware extends Middleware
{
private $requestPerMinute;
private $request;
private $currentTime;
public function __construct(int $requestPerMinute)
{
$this->requestPerMinute = $requestPerMinute;
$this->currentTime = time();
}
/**
* Please, note that the parent::check call can be inserted both at the
* beginning of this method and at the end.
*
* This gives much more flexibility than a simple loop over all middleware
* objects. For instance, a middleware can change the order of checks by
* running its check after all the others.
*/
public function check(string $email, string $password): bool
{
if (time() > $this->currentTime + 60) {
$this->request = 0;
$this->currentTime = time();
}
$this->request++;
if ($this->request > $this->requestPerMinute) {
echo "ThrottlingMiddleware: Request limit exceeded!\n";
die();
}
return parent::check($email, $password);
}
}
/**
* This is an application's class that acts as a real handler. The Server class
* uses the CoR pattern to execute a set of various authentication middleware
* before launching some business logic associated with a request.
*/
class Server
{
private $users = [];
/**
* @var Middleware
*/
private $middleware;
/**
* The client can configure the server with a chain of middleware objects.
*/
public function setMiddleware(Middleware $middleware): void
{
$this->middleware = $middleware;
}
/**
* The server gets the email and password from the client and sends the
* authorization request to the middleware.
*/
public function logIn(string $email, string $password): bool
{
if ($this->middleware->check($email, $password)) {
echo "Server: Authorization has been successful!\n";
// Do something useful for authorized users.
return true;
}
return false;
}
public function register(string $email, string $password): void
{
$this->users[$email] = $password;
}
public function hasEmail(string $email): bool
{
return isset($this->users[$email]);
}
public function isValidPassword(string $email, string $password): bool
{
return $this->users[$email] === $password;
}
}
/**
* The client code.
*/
$server = new Server();
$server->register("admin@example.com", "admin_pass");
$server->register("user@example.com", "user_pass");
// All middleware are chained. The client can build various configurations of
// chains depending on its needs.
$middleware = new ThrottlingMiddleware(2);
$middleware
->linkWith(new UserExistsMiddleware($server))
->linkWith(new RoleCheckMiddleware());
// The server gets a chain from the client code.
$server->setMiddleware($middleware);
// ...
do {
echo "\nEnter your email:\n";
$email = readline();
echo "Enter your password:\n";
$password = readline();
$success = $server->logIn($email, $password);
} while (!$success);
Output.txt: 실행 결과
Enter your email:
asd
Enter your password:
123
UserExistsMiddleware: This email is not registered!
Enter your email:
admin@example.com
Enter your password:
wrong
UserExistsMiddleware: Wrong password!
Enter your email:
admin@example.com
Enter your password:
letmein
ThrottlingMiddleware: Request limit exceeded!
Enter your email:
admin@example.com
Enter your password:
admin_pass
RoleCheckMiddleware: Hello, admin!
Server: Authorization has been successful!