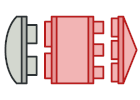
Adapter en Python
Adapter es un patrón de diseño estructural que permite colaborar a objetos incompatibles.
El patrón Adapter actúa como envoltorio entre dos objetos. Atrapa las llamadas a un objeto y las transforma a un formato y una interfaz reconocible para el segundo objeto.
Complejidad:
Popularidad:
Ejemplos de uso: El patrón Adapter es muy común en el código Python. Se utiliza muy a menudo en sistemas basados en algún código heredado. En estos casos, los adaptadores crean código heredado con clases modernas.
Identificación: Adapter es reconocible por un constructor que toma una instancia de distinto tipo de clase abstracta/interfaz. Cuando el adaptador recibe una llamada a uno de sus métodos, convierte los parámetros al formato adecuado y después dirige la llamada a uno o varios métodos del objeto envuelto.
Ejemplo conceptual
Este ejemplo ilustra la estructura del patrón de diseño Adapter. Se centra en responder las siguientes preguntas:
- ¿De qué clases se compone?
- ¿Qué papeles juegan esas clases?
- ¿De qué forma se relacionan los elementos del patrón?
main.py: Ejemplo conceptual
class Target:
"""
The Target defines the domain-specific interface used by the client code.
"""
def request(self) -> str:
return "Target: The default target's behavior."
class Adaptee:
"""
The Adaptee contains some useful behavior, but its interface is incompatible
with the existing client code. The Adaptee needs some adaptation before the
client code can use it.
"""
def specific_request(self) -> str:
return ".eetpadA eht fo roivaheb laicepS"
class Adapter(Target, Adaptee):
"""
The Adapter makes the Adaptee's interface compatible with the Target's
interface via multiple inheritance.
"""
def request(self) -> str:
return f"Adapter: (TRANSLATED) {self.specific_request()[::-1]}"
def client_code(target: "Target") -> None:
"""
The client code supports all classes that follow the Target interface.
"""
print(target.request(), end="")
if __name__ == "__main__":
print("Client: I can work just fine with the Target objects:")
target = Target()
client_code(target)
print("\n")
adaptee = Adaptee()
print("Client: The Adaptee class has a weird interface. "
"See, I don't understand it:")
print(f"Adaptee: {adaptee.specific_request()}", end="\n\n")
print("Client: But I can work with it via the Adapter:")
adapter = Adapter()
client_code(adapter)
Output.txt: Resultado de la ejecución
Client: I can work just fine with the Target objects:
Target: The default target's behavior.
Client: The Adaptee class has a weird interface. See, I don't understand it:
Adaptee: .eetpadA eht fo roivaheb laicepS
Client: But I can work with it via the Adapter:
Adapter: (TRANSLATED) Special behavior of the Adaptee.