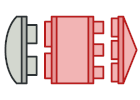
Adapter を Python で
Adapter は、 構造に関するデザインパターンの一つで、 非互換なオブジェクト同士の協働を可能とします。
アダプターは二つのオブジェクト間のラッパーとして機能します。 一方のオブジェクトへの呼び出しを捕まえ、 二つ目のオブジェクトが認識可能なデータ形式とインターフェースに変換します。
複雑度:
人気度:
使用例: Adapter パターンは Python コードではよく見かけます。 旧来のコードに基づいたシステムでよく使用されます。 そのような場合、 アダプターは旧来のコードを現代のクラスから使用可能にします。
見つけ方: アダプターは、 異なる抽象クラスまたはインターフェース型のインスタンスを取るコンストラクターの存在により認識できます。 アダプターは、 いずれかのメソッドへの呼び出しを受け取ると、 パラメーターを適切な形式に変換し、 ラップされたオブジェクトの一つまたは複数のメソッドを呼び出します。
概念的な例 (継承による)
この例は、 Adapter デザインパターンの構造を説明するためのものです。 以下の質問に答えることを目的としています:
- どういうクラスからできているか?
- それぞれのクラスの役割は?
- パターンの要素同士はどう関係しているのか?
main.py: 概念的な例
class Target:
"""
The Target defines the domain-specific interface used by the client code.
"""
def request(self) -> str:
return "Target: The default target's behavior."
class Adaptee:
"""
The Adaptee contains some useful behavior, but its interface is incompatible
with the existing client code. The Adaptee needs some adaptation before the
client code can use it.
"""
def specific_request(self) -> str:
return ".eetpadA eht fo roivaheb laicepS"
class Adapter(Target, Adaptee):
"""
The Adapter makes the Adaptee's interface compatible with the Target's
interface via multiple inheritance.
"""
def request(self) -> str:
return f"Adapter: (TRANSLATED) {self.specific_request()[::-1]}"
def client_code(target: "Target") -> None:
"""
The client code supports all classes that follow the Target interface.
"""
print(target.request(), end="")
if __name__ == "__main__":
print("Client: I can work just fine with the Target objects:")
target = Target()
client_code(target)
print("\n")
adaptee = Adaptee()
print("Client: The Adaptee class has a weird interface. "
"See, I don't understand it:")
print(f"Adaptee: {adaptee.specific_request()}", end="\n\n")
print("Client: But I can work with it via the Adapter:")
adapter = Adapter()
client_code(adapter)
Output.txt: 実行結果
Client: I can work just fine with the Target objects:
Target: The default target's behavior.
Client: The Adaptee class has a weird interface. See, I don't understand it:
Adaptee: .eetpadA eht fo roivaheb laicepS
Client: But I can work with it via the Adapter:
Adapter: (TRANSLATED) Special behavior of the Adaptee.
概念的な例(オブジェクト合成による)
この例は、 Adapter デザインパターンの構造を説明するためのものです。 以下の質問に答えることを目的としています:
- どういうクラスからできているか?
- それぞれのクラスの役割は?
- パターンの要素同士はどう関係しているのか?
main.py: 概念的な例
class Target:
"""
The Target defines the domain-specific interface used by the client code.
"""
def request(self) -> str:
return "Target: The default target's behavior."
class Adaptee:
"""
The Adaptee contains some useful behavior, but its interface is incompatible
with the existing client code. The Adaptee needs some adaptation before the
client code can use it.
"""
def specific_request(self) -> str:
return ".eetpadA eht fo roivaheb laicepS"
class Adapter(Target):
"""
The Adapter makes the Adaptee's interface compatible with the Target's
interface via composition.
"""
def __init__(self, adaptee: Adaptee) -> None:
self.adaptee = adaptee
def request(self) -> str:
return f"Adapter: (TRANSLATED) {self.adaptee.specific_request()[::-1]}"
def client_code(target: Target) -> None:
"""
The client code supports all classes that follow the Target interface.
"""
print(target.request(), end="")
if __name__ == "__main__":
print("Client: I can work just fine with the Target objects:")
target = Target()
client_code(target)
print("\n")
adaptee = Adaptee()
print("Client: The Adaptee class has a weird interface. "
"See, I don't understand it:")
print(f"Adaptee: {adaptee.specific_request()}", end="\n\n")
print("Client: But I can work with it via the Adapter:")
adapter = Adapter(adaptee)
client_code(adapter)
Output.txt: 実行結果
Client: I can work just fine with the Target objects:
Target: The default target's behavior.
Client: The Adaptee class has a weird interface. See, I don't understand it:
Adaptee: .eetpadA eht fo roivaheb laicepS
Client: But I can work with it via the Adapter:
Adapter: (TRANSLATED) Special behavior of the Adaptee.