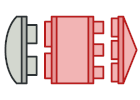
Adapter を Go で
Adapter は、 構造に関するデザインパターンの一つで、 非互換なオブジェクト同士の協働を可能とします。
アダプターは二つのオブジェクト間のラッパーとして機能します。 一方のオブジェクトへの呼び出しを捕まえ、 二つ目のオブジェクトが認識可能なデータ形式とインターフェースに変換します。
概念的な例
オブジェクトの何らかの機能 (ライトニング・ポート) の存在を期待するクライアント・コードがあります。 しかし、 ここで adaptee と呼ばれるオブジェクト (Windows ラップトップ) は、 同じ機能を異なるインターフェース (USB ポート) を通して提供します。
ここで Adapter パターンが登場します。 以下を行う adapter という struct 型を作成します:
-
クライアントが期待しているのと同じインターフェース (ライトニング・ポート) に従う。
-
クライアントからのリクエストを adaptee が期待する形式に翻訳する。 adapter は、 ライトニング・コネクターを受け入れ、 その信号を USB 形式に変換し、 Windows ラップトップの USB ポートに渡す。
client.go: クライアント・コード
package main
import "fmt"
type Client struct {
}
func (c *Client) InsertLightningConnectorIntoComputer(com Computer) {
fmt.Println("Client inserts Lightning connector into computer.")
com.InsertIntoLightningPort()
}
computer.go: クライアント・インターフェース
package main
type Computer interface {
InsertIntoLightningPort()
}
mac.go: サービス
package main
import "fmt"
type Mac struct {
}
func (m *Mac) InsertIntoLightningPort() {
fmt.Println("Lightning connector is plugged into mac machine.")
}
windows.go: 不明なサービス
package main
import "fmt"
type Windows struct{}
func (w *Windows) insertIntoUSBPort() {
fmt.Println("USB connector is plugged into windows machine.")
}
windowsAdapter.go: アダプター
package main
import "fmt"
type WindowsAdapter struct {
windowMachine *Windows
}
func (w *WindowsAdapter) InsertIntoLightningPort() {
fmt.Println("Adapter converts Lightning signal to USB.")
w.windowMachine.insertIntoUSBPort()
}
main.go
package main
func main() {
client := &Client{}
mac := &Mac{}
client.InsertLightningConnectorIntoComputer(mac)
windowsMachine := &Windows{}
windowsMachineAdapter := &WindowsAdapter{
windowMachine: windowsMachine,
}
client.InsertLightningConnectorIntoComputer(windowsMachineAdapter)
}
output.txt: 実行結果
Client inserts Lightning connector into computer.
Lightning connector is plugged into mac machine.
Client inserts Lightning connector into computer.
Adapter converts Lightning signal to USB.
USB connector is plugged into windows machine.