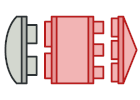
Adapter を C# で
Adapter は、 構造に関するデザインパターンの一つで、 非互換なオブジェクト同士の協働を可能とします。
アダプターは二つのオブジェクト間のラッパーとして機能します。 一方のオブジェクトへの呼び出しを捕まえ、 二つ目のオブジェクトが認識可能なデータ形式とインターフェースに変換します。
複雑度:
人気度:
使用例: Adapter パターンは C# コードではよく見かけます。 旧来のコードに基づいたシステムでよく使用されます。 そのような場合、 アダプターは旧来のコードを現代のクラスから使用可能にします。
見つけ方: アダプターは、 異なる抽象クラスまたはインターフェース型のインスタンスを取るコンストラクターの存在により認識できます。 アダプターは、 いずれかのメソッドへの呼び出しを受け取ると、 パラメーターを適切な形式に変換し、 ラップされたオブジェクトの一つまたは複数のメソッドを呼び出します。
概念的な例
この例は、 Adapter デザインパターンの構造を説明するためのものです。 以下の質問に答えることを目的としています:
- どういうクラスからできているか?
- それぞれのクラスの役割は?
- パターンの要素同士はどう関係しているのか?
Program.cs: 概念的な例
using System;
namespace RefactoringGuru.DesignPatterns.Adapter.Conceptual
{
// The Target defines the domain-specific interface used by the client code.
public interface ITarget
{
string GetRequest();
}
// The Adaptee contains some useful behavior, but its interface is
// incompatible with the existing client code. The Adaptee needs some
// adaptation before the client code can use it.
class Adaptee
{
public string GetSpecificRequest()
{
return "Specific request.";
}
}
// The Adapter makes the Adaptee's interface compatible with the Target's
// interface.
class Adapter : ITarget
{
private readonly Adaptee _adaptee;
public Adapter(Adaptee adaptee)
{
this._adaptee = adaptee;
}
public string GetRequest()
{
return $"This is '{this._adaptee.GetSpecificRequest()}'";
}
}
class Program
{
static void Main(string[] args)
{
Adaptee adaptee = new Adaptee();
ITarget target = new Adapter(adaptee);
Console.WriteLine("Adaptee interface is incompatible with the client.");
Console.WriteLine("But with adapter client can call it's method.");
Console.WriteLine(target.GetRequest());
}
}
}
Output.txt: 実行結果
Adaptee interface is incompatible with the client.
But with adapter client can call it's method.
This is 'Specific request.'