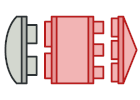
Adapter w języku Python
Adapter to strukturalny wzorzec projektowy pozwalający na współpracę niekompatybilnych obiektów ze sobą.
Adapter pełni rolę opakowania dwóch obiektów. Przechwytuje wywołania jednego z obiektów i przekształca je na format i interfejs zrozumiały dla drugiego obiektu.
Złożoność:
Popularność:
Przykłady użycia: Wzorzec Adapter jest dość powszechny w kodzie Python. Często stosuje się go w systemach bazujących na przestarzałym kodzie, gdzie pozwala na współdziałanie takiego kodu z nowoczesnymi klasami.
Identyfikacja: Adapter można rozpoznać po konstruktorze przyjmującym instancję innego typu abstrakcji/interfejsu. Gdy adapter otrzymuje wywołanie kierowane do którejś z jego metod, tłumaczy parametry wywołania do stosownego formatu i przekazuje je do jednej lub wielu metod opakowanego obiektu.
Przykład koncepcyjny
Poniższy przykład ilustruje strukturę wzorca Adapter ze szczególnym naciskiem na następujące kwestie:
- Z jakich składa się klas?
- Jakie role pełnią te klasy?
- W jaki sposób elementy wzorca są ze sobą powiązane?
main.py: Przykład koncepcyjny
class Target:
"""
The Target defines the domain-specific interface used by the client code.
"""
def request(self) -> str:
return "Target: The default target's behavior."
class Adaptee:
"""
The Adaptee contains some useful behavior, but its interface is incompatible
with the existing client code. The Adaptee needs some adaptation before the
client code can use it.
"""
def specific_request(self) -> str:
return ".eetpadA eht fo roivaheb laicepS"
class Adapter(Target, Adaptee):
"""
The Adapter makes the Adaptee's interface compatible with the Target's
interface via multiple inheritance.
"""
def request(self) -> str:
return f"Adapter: (TRANSLATED) {self.specific_request()[::-1]}"
def client_code(target: "Target") -> None:
"""
The client code supports all classes that follow the Target interface.
"""
print(target.request(), end="")
if __name__ == "__main__":
print("Client: I can work just fine with the Target objects:")
target = Target()
client_code(target)
print("\n")
adaptee = Adaptee()
print("Client: The Adaptee class has a weird interface. "
"See, I don't understand it:")
print(f"Adaptee: {adaptee.specific_request()}", end="\n\n")
print("Client: But I can work with it via the Adapter:")
adapter = Adapter()
client_code(adapter)
Output.txt: Wynik działania
Client: I can work just fine with the Target objects:
Target: The default target's behavior.
Client: The Adaptee class has a weird interface. See, I don't understand it:
Adaptee: .eetpadA eht fo roivaheb laicepS
Client: But I can work with it via the Adapter:
Adapter: (TRANSLATED) Special behavior of the Adaptee.