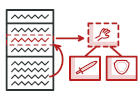
Strategy を C++ で
Strategy は、 振る舞いに関するデザインパターンの一つで、 一連の振る舞いをオブジェクトに転換し、 元のコンテキスト・オブジェクト内で交換可能とします。
元のオブジェクトは、 コンテキストと呼ばれ、 一つのストラテジー・オブジェクトへの参照を保持し、 それに振る舞いの実行を委任します。 コンテキストがその作業を実行する方法を変えるために、 他のオブジェクトが、 現在リンクされているオブジェクトを違うものと置き換えるかもしれません。
複雑度:
人気度:
使用例: Strategy パターンは、 C++ コードではよく見かけます。 種々のフレームワークで、 クラスの拡張をせずに、 その振る舞いを変更できるようにするためによく使われます。
見つけ方: 入れ子になったオブジェクトに何か実際の作業をさせるメソッドや、 そのオブジェクトを他のものと入れ替えるための setter の存在で、 Strategy パターンを識別できます。
概念的な例
この例は、 Strategy デザインパターンの構造を説明するためのものです。 以下の質問に答えることを目的としています:
- どういうクラスからできているか?
- それぞれのクラスの役割は?
- パターンの要素同士はどう関係しているのか?
main.cc: 概念的な例
/**
* The Strategy interface declares operations common to all supported versions
* of some algorithm.
*
* The Context uses this interface to call the algorithm defined by Concrete
* Strategies.
*/
class Strategy
{
public:
virtual ~Strategy() = default;
virtual std::string doAlgorithm(std::string_view data) const = 0;
};
/**
* The Context defines the interface of interest to clients.
*/
class Context
{
/**
* @var Strategy The Context maintains a reference to one of the Strategy
* objects. The Context does not know the concrete class of a strategy. It
* should work with all strategies via the Strategy interface.
*/
private:
std::unique_ptr<Strategy> strategy_;
/**
* Usually, the Context accepts a strategy through the constructor, but also
* provides a setter to change it at runtime.
*/
public:
explicit Context(std::unique_ptr<Strategy> &&strategy = {}) : strategy_(std::move(strategy))
{
}
/**
* Usually, the Context allows replacing a Strategy object at runtime.
*/
void set_strategy(std::unique_ptr<Strategy> &&strategy)
{
strategy_ = std::move(strategy);
}
/**
* The Context delegates some work to the Strategy object instead of
* implementing +multiple versions of the algorithm on its own.
*/
void doSomeBusinessLogic() const
{
if (strategy_) {
std::cout << "Context: Sorting data using the strategy (not sure how it'll do it)\n";
std::string result = strategy_->doAlgorithm("aecbd");
std::cout << result << "\n";
} else {
std::cout << "Context: Strategy isn't set\n";
}
}
};
/**
* Concrete Strategies implement the algorithm while following the base Strategy
* interface. The interface makes them interchangeable in the Context.
*/
class ConcreteStrategyA : public Strategy
{
public:
std::string doAlgorithm(std::string_view data) const override
{
std::string result(data);
std::sort(std::begin(result), std::end(result));
return result;
}
};
class ConcreteStrategyB : public Strategy
{
std::string doAlgorithm(std::string_view data) const override
{
std::string result(data);
std::sort(std::begin(result), std::end(result), std::greater<>());
return result;
}
};
/**
* The client code picks a concrete strategy and passes it to the context. The
* client should be aware of the differences between strategies in order to make
* the right choice.
*/
void clientCode()
{
Context context(std::make_unique<ConcreteStrategyA>());
std::cout << "Client: Strategy is set to normal sorting.\n";
context.doSomeBusinessLogic();
std::cout << "\n";
std::cout << "Client: Strategy is set to reverse sorting.\n";
context.set_strategy(std::make_unique<ConcreteStrategyB>());
context.doSomeBusinessLogic();
}
int main()
{
clientCode();
return 0;
}
Output.txt: 実行結果
Client: Strategy is set to normal sorting.
Context: Sorting data using the strategy (not sure how it'll do it)
abcde
Client: Strategy is set to reverse sorting.
Context: Sorting data using the strategy (not sure how it'll do it)
edcba