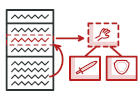
Strategy を C# で
Strategy は、 振る舞いに関するデザインパターンの一つで、 一連の振る舞いをオブジェクトに転換し、 元のコンテキスト・オブジェクト内で交換可能とします。
元のオブジェクトは、 コンテキストと呼ばれ、 一つのストラテジー・オブジェクトへの参照を保持し、 それに振る舞いの実行を委任します。 コンテキストがその作業を実行する方法を変えるために、 他のオブジェクトが、 現在リンクされているオブジェクトを違うものと置き換えるかもしれません。
複雑度:
人気度:
使用例: Strategy パターンは、 C# コードではよく見かけます。 種々のフレームワークで、 クラスの拡張をせずに、 その振る舞いを変更できるようにするためによく使われます。
見つけ方: 入れ子になったオブジェクトに何か実際の作業をさせるメソッドや、 そのオブジェクトを他のものと入れ替えるための setter の存在で、 Strategy パターンを識別できます。
概念的な例
この例は、 Strategy デザインパターンの構造を説明するためのものです。 以下の質問に答えることを目的としています:
- どういうクラスからできているか?
- それぞれのクラスの役割は?
- パターンの要素同士はどう関係しているのか?
Program.cs: 概念的な例
using System;
using System.Collections.Generic;
namespace RefactoringGuru.DesignPatterns.Strategy.Conceptual
{
// The Context defines the interface of interest to clients.
class Context
{
// The Context maintains a reference to one of the Strategy objects. The
// Context does not know the concrete class of a strategy. It should
// work with all strategies via the Strategy interface.
private IStrategy _strategy;
public Context()
{ }
// Usually, the Context accepts a strategy through the constructor, but
// also provides a setter to change it at runtime.
public Context(IStrategy strategy)
{
this._strategy = strategy;
}
// Usually, the Context allows replacing a Strategy object at runtime.
public void SetStrategy(IStrategy strategy)
{
this._strategy = strategy;
}
// The Context delegates some work to the Strategy object instead of
// implementing multiple versions of the algorithm on its own.
public void DoSomeBusinessLogic()
{
Console.WriteLine("Context: Sorting data using the strategy (not sure how it'll do it)");
var result = this._strategy.DoAlgorithm(new List<string> { "a", "b", "c", "d", "e" });
string resultStr = string.Empty;
foreach (var element in result as List<string>)
{
resultStr += element + ",";
}
Console.WriteLine(resultStr);
}
}
// The Strategy interface declares operations common to all supported
// versions of some algorithm.
//
// The Context uses this interface to call the algorithm defined by Concrete
// Strategies.
public interface IStrategy
{
object DoAlgorithm(object data);
}
// Concrete Strategies implement the algorithm while following the base
// Strategy interface. The interface makes them interchangeable in the
// Context.
class ConcreteStrategyA : IStrategy
{
public object DoAlgorithm(object data)
{
var list = data as List<string>;
list.Sort();
return list;
}
}
class ConcreteStrategyB : IStrategy
{
public object DoAlgorithm(object data)
{
var list = data as List<string>;
list.Sort();
list.Reverse();
return list;
}
}
class Program
{
static void Main(string[] args)
{
// The client code picks a concrete strategy and passes it to the
// context. The client should be aware of the differences between
// strategies in order to make the right choice.
var context = new Context();
Console.WriteLine("Client: Strategy is set to normal sorting.");
context.SetStrategy(new ConcreteStrategyA());
context.DoSomeBusinessLogic();
Console.WriteLine();
Console.WriteLine("Client: Strategy is set to reverse sorting.");
context.SetStrategy(new ConcreteStrategyB());
context.DoSomeBusinessLogic();
}
}
}
Output.txt: 実行結果
Client: Strategy is set to normal sorting.
Context: Sorting data using the strategy (not sure how it'll do it)
a,b,c,d,e
Client: Strategy is set to reverse sorting.
Context: Sorting data using the strategy (not sure how it'll do it)
e,d,c,b,a