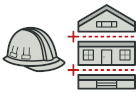
C#으로 작성된 빌더
빌더는 복잡한 객체들을 단계별로 생성할 수 있도록 하는 생성 디자인 패턴입니다.
다른 생성 패턴과 달리 빌더 패턴은 제품들에 공통 인터페이스를 요구하지 않습니다. 이를 통해 같은 생성공정을 사용하여 다양한 제품을 생산할 수 있습니다.
복잡도:
인기도:
사용 예시들: 빌더 패턴은 C 개발자들에게 잘 알려진 패턴이며, 가능한 설정 옵션이 많은 객체를 만들어야 할 때 특히 유용합니다.
식별법: 빌더 패턴은 하나의 생성 메서드와 결과 객체를 설정하기 위한 여러 메서드가 있는 클래스가 있습니다. 또 빌더 메서드들은 자주 사슬식 연결을 지원합니다 (예: someBuilder.setValueA(1).setValueB(2).create()
).
개념적인 예시
이 예시는 빌더 디자인 패턴의 구조를 보여주고 다음 질문에 중점을 둡니다:
- 패턴은 어떤 클래스들로 구성되어 있나요?
- 이 클래스들은 어떤 역할을 하나요?
- 패턴의 요소들은 어떻게 서로 연관되어 있나요?
Program.cs: 개념적인 예시
using System;
using System.Collections.Generic;
namespace RefactoringGuru.DesignPatterns.Builder.Conceptual
{
// The Builder interface specifies methods for creating the different parts
// of the Product objects.
public interface IBuilder
{
void BuildPartA();
void BuildPartB();
void BuildPartC();
}
// The Concrete Builder classes follow the Builder interface and provide
// specific implementations of the building steps. Your program may have
// several variations of Builders, implemented differently.
public class ConcreteBuilder : IBuilder
{
private Product _product = new Product();
// A fresh builder instance should contain a blank product object, which
// is used in further assembly.
public ConcreteBuilder()
{
this.Reset();
}
public void Reset()
{
this._product = new Product();
}
// All production steps work with the same product instance.
public void BuildPartA()
{
this._product.Add("PartA1");
}
public void BuildPartB()
{
this._product.Add("PartB1");
}
public void BuildPartC()
{
this._product.Add("PartC1");
}
// Concrete Builders are supposed to provide their own methods for
// retrieving results. That's because various types of builders may
// create entirely different products that don't follow the same
// interface. Therefore, such methods cannot be declared in the base
// Builder interface (at least in a statically typed programming
// language).
//
// Usually, after returning the end result to the client, a builder
// instance is expected to be ready to start producing another product.
// That's why it's a usual practice to call the reset method at the end
// of the `GetProduct` method body. However, this behavior is not
// mandatory, and you can make your builders wait for an explicit reset
// call from the client code before disposing of the previous result.
public Product GetProduct()
{
Product result = this._product;
this.Reset();
return result;
}
}
// It makes sense to use the Builder pattern only when your products are
// quite complex and require extensive configuration.
//
// Unlike in other creational patterns, different concrete builders can
// produce unrelated products. In other words, results of various builders
// may not always follow the same interface.
public class Product
{
private List<object> _parts = new List<object>();
public void Add(string part)
{
this._parts.Add(part);
}
public string ListParts()
{
string str = string.Empty;
for (int i = 0; i < this._parts.Count; i++)
{
str += this._parts[i] + ", ";
}
str = str.Remove(str.Length - 2); // removing last ",c"
return "Product parts: " + str + "\n";
}
}
// The Director is only responsible for executing the building steps in a
// particular sequence. It is helpful when producing products according to a
// specific order or configuration. Strictly speaking, the Director class is
// optional, since the client can control builders directly.
public class Director
{
private IBuilder _builder;
public IBuilder Builder
{
set { _builder = value; }
}
// The Director can construct several product variations using the same
// building steps.
public void BuildMinimalViableProduct()
{
this._builder.BuildPartA();
}
public void BuildFullFeaturedProduct()
{
this._builder.BuildPartA();
this._builder.BuildPartB();
this._builder.BuildPartC();
}
}
class Program
{
static void Main(string[] args)
{
// The client code creates a builder object, passes it to the
// director and then initiates the construction process. The end
// result is retrieved from the builder object.
var director = new Director();
var builder = new ConcreteBuilder();
director.Builder = builder;
Console.WriteLine("Standard basic product:");
director.BuildMinimalViableProduct();
Console.WriteLine(builder.GetProduct().ListParts());
Console.WriteLine("Standard full featured product:");
director.BuildFullFeaturedProduct();
Console.WriteLine(builder.GetProduct().ListParts());
// Remember, the Builder pattern can be used without a Director
// class.
Console.WriteLine("Custom product:");
builder.BuildPartA();
builder.BuildPartC();
Console.Write(builder.GetProduct().ListParts());
}
}
}
Output.txt: 실행 결과
Standard basic product:
Product parts: PartA1
Standard full featured product:
Product parts: PartA1, PartB1, PartC1
Custom product:
Product parts: PartA1, PartC1