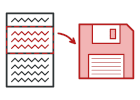
자바로 작성된 메멘토
메멘토 패턴은 행동 디자인 패턴입니다. 이 패턴은 객체 상태의 스냅숏을 만든 후 나중에 복원할 수 있도록 합니다.
메멘토는 함께 작동하는 객체의 내부 구조와 스냅숏들 내부에 보관된 데이터를 손상하지 않습니다.
복잡도:
인기도:
사용 사례들: 메멘토의 원칙은 직렬화를 사용하여 달성할 수 있으며, 이는 자바에서 매우 일반적입니다. 직렬화는 객체 상태의 스냅숏을 만드는 유일한 또는 가장 효율적인 방법은 아니나 다른 객체로부터 오리지네이터의 구조를 보호하면서 상태 백업을 저장할 수 있도록 합니다.
다음은 코어 자바 라이브러리로부터 가져온 패턴의 몇 가지 예시들입니다:
- 모든
java.io.Serializable
구현들은 메멘토를 시뮬레이션할 수 있습니다. - 모든
javax.faces.component.StateHolder
구현들
모양 편집기와 복잡한 실행 취소/다시 실행
이 그래픽 편집기를 사용하면 화면에서 모양의 색상과 위치를 변경할 수 있으며, 또 모든 수정을 취소 또는 반복할 수 있습니다.
'실행 취소'는 메멘토와 커맨드 패턴 간의 협업을 기반으로 합니다. 편집기는 수행된 커맨드들의 기록을 추적합니다. 커맨드를 실행하기 전에 편집기는 백업을 만든 후 커맨드 객체에 연결합니다. 커맨드를 실행 후 편집기는 커맨드를 기록으로 푸시합니다.
사용자가 실행 취소를 요청하면 편집기는 기록에서 최근 명령을 가져온 후 해당 명령 내부에 보관된 백업에서 상태를 복원합니다. 사용자가 다른 실행 취소를 요청하면 편집기는 다음 명령을 기록에서 가져옵니다.
실행 취소된 명령들은 사용자가 화면의 모양들을 수정할 때까지 기록에 보관됩니다. 이것은 실행 취소된 명령을 다시 실행하는 데 중요합니다.
editor
editor/Editor.java: 편집자 코드
package refactoring_guru.memento.example.editor;
import refactoring_guru.memento.example.commands.Command;
import refactoring_guru.memento.example.history.History;
import refactoring_guru.memento.example.history.Memento;
import refactoring_guru.memento.example.shapes.CompoundShape;
import refactoring_guru.memento.example.shapes.Shape;
import javax.swing.*;
import java.io.*;
import java.util.Base64;
public class Editor extends JComponent {
private Canvas canvas;
private CompoundShape allShapes = new CompoundShape();
private History history;
public Editor() {
canvas = new Canvas(this);
history = new History();
}
public void loadShapes(Shape... shapes) {
allShapes.clear();
allShapes.add(shapes);
canvas.refresh();
}
public CompoundShape getShapes() {
return allShapes;
}
public void execute(Command c) {
history.push(c, new Memento(this));
c.execute();
}
public void undo() {
if (history.undo())
canvas.repaint();
}
public void redo() {
if (history.redo())
canvas.repaint();
}
public String backup() {
try {
ByteArrayOutputStream baos = new ByteArrayOutputStream();
ObjectOutputStream oos = new ObjectOutputStream(baos);
oos.writeObject(this.allShapes);
oos.close();
return Base64.getEncoder().encodeToString(baos.toByteArray());
} catch (IOException e) {
return "";
}
}
public void restore(String state) {
try {
byte[] data = Base64.getDecoder().decode(state);
ObjectInputStream ois = new ObjectInputStream(new ByteArrayInputStream(data));
this.allShapes = (CompoundShape) ois.readObject();
ois.close();
} catch (ClassNotFoundException e) {
System.out.print("ClassNotFoundException occurred.");
} catch (IOException e) {
System.out.print("IOException occurred.");
}
}
}
editor/Canvas.java: 캔버스 코드
package refactoring_guru.memento.example.editor;
import refactoring_guru.memento.example.commands.ColorCommand;
import refactoring_guru.memento.example.commands.MoveCommand;
import refactoring_guru.memento.example.shapes.Shape;
import javax.swing.*;
import javax.swing.border.Border;
import java.awt.*;
import java.awt.event.*;
import java.awt.image.BufferedImage;
class Canvas extends java.awt.Canvas {
private Editor editor;
private JFrame frame;
private static final int PADDING = 10;
Canvas(Editor editor) {
this.editor = editor;
createFrame();
attachKeyboardListeners();
attachMouseListeners();
refresh();
}
private void createFrame() {
frame = new JFrame();
frame.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
frame.setLocationRelativeTo(null);
JPanel contentPanel = new JPanel();
Border padding = BorderFactory.createEmptyBorder(PADDING, PADDING, PADDING, PADDING);
contentPanel.setBorder(padding);
contentPanel.setLayout(new BoxLayout(contentPanel, BoxLayout.Y_AXIS));
frame.setContentPane(contentPanel);
contentPanel.add(new JLabel("Select and drag to move."), BorderLayout.PAGE_END);
contentPanel.add(new JLabel("Right click to change color."), BorderLayout.PAGE_END);
contentPanel.add(new JLabel("Undo: Ctrl+Z, Redo: Ctrl+R"), BorderLayout.PAGE_END);
contentPanel.add(this);
frame.setVisible(true);
contentPanel.setBackground(Color.LIGHT_GRAY);
}
private void attachKeyboardListeners() {
addKeyListener(new KeyAdapter() {
@Override
public void keyPressed(KeyEvent e) {
if ((e.getModifiers() & KeyEvent.CTRL_MASK) != 0) {
switch (e.getKeyCode()) {
case KeyEvent.VK_Z:
editor.undo();
break;
case KeyEvent.VK_R:
editor.redo();
break;
}
}
}
});
}
private void attachMouseListeners() {
MouseAdapter colorizer = new MouseAdapter() {
@Override
public void mousePressed(MouseEvent e) {
if (e.getButton() != MouseEvent.BUTTON3) {
return;
}
Shape target = editor.getShapes().getChildAt(e.getX(), e.getY());
if (target != null) {
editor.execute(new ColorCommand(editor, new Color((int) (Math.random() * 0x1000000))));
repaint();
}
}
};
addMouseListener(colorizer);
MouseAdapter selector = new MouseAdapter() {
@Override
public void mousePressed(MouseEvent e) {
if (e.getButton() != MouseEvent.BUTTON1) {
return;
}
Shape target = editor.getShapes().getChildAt(e.getX(), e.getY());
boolean ctrl = (e.getModifiers() & ActionEvent.CTRL_MASK) == ActionEvent.CTRL_MASK;
if (target == null) {
if (!ctrl) {
editor.getShapes().unSelect();
}
} else {
if (ctrl) {
if (target.isSelected()) {
target.unSelect();
} else {
target.select();
}
} else {
if (!target.isSelected()) {
editor.getShapes().unSelect();
}
target.select();
}
}
repaint();
}
};
addMouseListener(selector);
MouseAdapter dragger = new MouseAdapter() {
MoveCommand moveCommand;
@Override
public void mouseDragged(MouseEvent e) {
if ((e.getModifiersEx() & MouseEvent.BUTTON1_DOWN_MASK) != MouseEvent.BUTTON1_DOWN_MASK) {
return;
}
if (moveCommand == null) {
moveCommand = new MoveCommand(editor);
moveCommand.start(e.getX(), e.getY());
}
moveCommand.move(e.getX(), e.getY());
repaint();
}
@Override
public void mouseReleased(MouseEvent e) {
if (e.getButton() != MouseEvent.BUTTON1 || moveCommand == null) {
return;
}
moveCommand.stop(e.getX(), e.getY());
editor.execute(moveCommand);
this.moveCommand = null;
repaint();
}
};
addMouseListener(dragger);
addMouseMotionListener(dragger);
}
public int getWidth() {
return editor.getShapes().getX() + editor.getShapes().getWidth() + PADDING;
}
public int getHeight() {
return editor.getShapes().getY() + editor.getShapes().getHeight() + PADDING;
}
void refresh() {
this.setSize(getWidth(), getHeight());
frame.pack();
}
public void update(Graphics g) {
paint(g);
}
public void paint(Graphics graphics) {
BufferedImage buffer = new BufferedImage(this.getWidth(), this.getHeight(), BufferedImage.TYPE_INT_RGB);
Graphics2D ig2 = buffer.createGraphics();
ig2.setBackground(Color.WHITE);
ig2.clearRect(0, 0, this.getWidth(), this.getHeight());
editor.getShapes().paint(buffer.getGraphics());
graphics.drawImage(buffer, 0, 0, null);
}
}
history
history/History.java: 기록은 커맨드들과 메멘토들을 저장합니다
package refactoring_guru.memento.example.history;
import refactoring_guru.memento.example.commands.Command;
import java.util.ArrayList;
import java.util.List;
public class History {
private List<Pair> history = new ArrayList<Pair>();
private int virtualSize = 0;
private class Pair {
Command command;
Memento memento;
Pair(Command c, Memento m) {
command = c;
memento = m;
}
private Command getCommand() {
return command;
}
private Memento getMemento() {
return memento;
}
}
public void push(Command c, Memento m) {
if (virtualSize != history.size() && virtualSize > 0) {
history = history.subList(0, virtualSize - 1);
}
history.add(new Pair(c, m));
virtualSize = history.size();
}
public boolean undo() {
Pair pair = getUndo();
if (pair == null) {
return false;
}
System.out.println("Undoing: " + pair.getCommand().getName());
pair.getMemento().restore();
return true;
}
public boolean redo() {
Pair pair = getRedo();
if (pair == null) {
return false;
}
System.out.println("Redoing: " + pair.getCommand().getName());
pair.getMemento().restore();
pair.getCommand().execute();
return true;
}
private Pair getUndo() {
if (virtualSize == 0) {
return null;
}
virtualSize = Math.max(0, virtualSize - 1);
return history.get(virtualSize);
}
private Pair getRedo() {
if (virtualSize == history.size()) {
return null;
}
virtualSize = Math.min(history.size(), virtualSize + 1);
return history.get(virtualSize - 1);
}
}
history/Memento.java: 메멘토 클래스
package refactoring_guru.memento.example.history;
import refactoring_guru.memento.example.editor.Editor;
public class Memento {
private String backup;
private Editor editor;
public Memento(Editor editor) {
this.editor = editor;
this.backup = editor.backup();
}
public void restore() {
editor.restore(backup);
}
}
commands
commands/Command.java: 기초 커맨드 클래스
package refactoring_guru.memento.example.commands;
public interface Command {
String getName();
void execute();
}
commands/ColorCommand.java: 선택된 모양의 색상 변경
package refactoring_guru.memento.example.commands;
import refactoring_guru.memento.example.editor.Editor;
import refactoring_guru.memento.example.shapes.Shape;
import java.awt.*;
public class ColorCommand implements Command {
private Editor editor;
private Color color;
public ColorCommand(Editor editor, Color color) {
this.editor = editor;
this.color = color;
}
@Override
public String getName() {
return "Colorize: " + color.toString();
}
@Override
public void execute() {
for (Shape child : editor.getShapes().getSelected()) {
child.setColor(color);
}
}
}
commands/MoveCommand.java: 선택된 모양을 이동합니다
package refactoring_guru.memento.example.commands;
import refactoring_guru.memento.example.editor.Editor;
import refactoring_guru.memento.example.shapes.Shape;
public class MoveCommand implements Command {
private Editor editor;
private int startX, startY;
private int endX, endY;
public MoveCommand(Editor editor) {
this.editor = editor;
}
@Override
public String getName() {
return "Move by X:" + (endX - startX) + " Y:" + (endY - startY);
}
public void start(int x, int y) {
startX = x;
startY = y;
for (Shape child : editor.getShapes().getSelected()) {
child.drag();
}
}
public void move(int x, int y) {
for (Shape child : editor.getShapes().getSelected()) {
child.moveTo(x - startX, y - startY);
}
}
public void stop(int x, int y) {
endX = x;
endY = y;
for (Shape child : editor.getShapes().getSelected()) {
child.drop();
}
}
@Override
public void execute() {
for (Shape child : editor.getShapes().getSelected()) {
child.moveBy(endX - startX, endY - startY);
}
}
}
shapes: 다양한 모양들
shapes/Shape.java
package refactoring_guru.memento.example.shapes;
import java.awt.*;
import java.io.Serializable;
public interface Shape extends Serializable {
int getX();
int getY();
int getWidth();
int getHeight();
void drag();
void drop();
void moveTo(int x, int y);
void moveBy(int x, int y);
boolean isInsideBounds(int x, int y);
Color getColor();
void setColor(Color color);
void select();
void unSelect();
boolean isSelected();
void paint(Graphics graphics);
}
shapes/BaseShape.java
package refactoring_guru.memento.example.shapes;
import java.awt.*;
public abstract class BaseShape implements Shape {
int x, y;
private int dx = 0, dy = 0;
private Color color;
private boolean selected = false;
BaseShape(int x, int y, Color color) {
this.x = x;
this.y = y;
this.color = color;
}
@Override
public int getX() {
return x;
}
@Override
public int getY() {
return y;
}
@Override
public int getWidth() {
return 0;
}
@Override
public int getHeight() {
return 0;
}
@Override
public void drag() {
dx = x;
dy = y;
}
@Override
public void moveTo(int x, int y) {
this.x = dx + x;
this.y = dy + y;
}
@Override
public void moveBy(int x, int y) {
this.x += x;
this.y += y;
}
@Override
public void drop() {
this.x = dx;
this.y = dy;
}
@Override
public boolean isInsideBounds(int x, int y) {
return x > getX() && x < (getX() + getWidth()) &&
y > getY() && y < (getY() + getHeight());
}
@Override
public Color getColor() {
return color;
}
@Override
public void setColor(Color color) {
this.color = color;
}
@Override
public void select() {
selected = true;
}
@Override
public void unSelect() {
selected = false;
}
@Override
public boolean isSelected() {
return selected;
}
void enableSelectionStyle(Graphics graphics) {
graphics.setColor(Color.LIGHT_GRAY);
Graphics2D g2 = (Graphics2D) graphics;
float[] dash1 = {2.0f};
g2.setStroke(new BasicStroke(1.0f,
BasicStroke.CAP_BUTT,
BasicStroke.JOIN_MITER,
2.0f, dash1, 0.0f));
}
void disableSelectionStyle(Graphics graphics) {
graphics.setColor(color);
Graphics2D g2 = (Graphics2D) graphics;
g2.setStroke(new BasicStroke());
}
@Override
public void paint(Graphics graphics) {
if (isSelected()) {
enableSelectionStyle(graphics);
}
else {
disableSelectionStyle(graphics);
}
// ...
}
}
shapes/Circle.java
package refactoring_guru.memento.example.shapes;
import java.awt.*;
public class Circle extends BaseShape {
private int radius;
public Circle(int x, int y, int radius, Color color) {
super(x, y, color);
this.radius = radius;
}
@Override
public int getWidth() {
return radius * 2;
}
@Override
public int getHeight() {
return radius * 2;
}
@Override
public void paint(Graphics graphics) {
super.paint(graphics);
graphics.drawOval(x, y, getWidth() - 1, getHeight() - 1);
}
}
shapes/Dot.java
package refactoring_guru.memento.example.shapes;
import java.awt.*;
public class Dot extends BaseShape {
private final int DOT_SIZE = 3;
public Dot(int x, int y, Color color) {
super(x, y, color);
}
@Override
public int getWidth() {
return DOT_SIZE;
}
@Override
public int getHeight() {
return DOT_SIZE;
}
@Override
public void paint(Graphics graphics) {
super.paint(graphics);
graphics.fillRect(x - 1, y - 1, getWidth(), getHeight());
}
}
shapes/Rectangle.java
package refactoring_guru.memento.example.shapes;
import java.awt.*;
public class Rectangle extends BaseShape {
private int width;
private int height;
public Rectangle(int x, int y, int width, int height, Color color) {
super(x, y, color);
this.width = width;
this.height = height;
}
@Override
public int getWidth() {
return width;
}
@Override
public int getHeight() {
return height;
}
@Override
public void paint(Graphics graphics) {
super.paint(graphics);
graphics.drawRect(x, y, getWidth() - 1, getHeight() - 1);
}
}
shapes/CompoundShape.java
package refactoring_guru.memento.example.shapes;
import java.awt.*;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
public class CompoundShape extends BaseShape {
private List<Shape> children = new ArrayList<>();
public CompoundShape(Shape... components) {
super(0, 0, Color.BLACK);
add(components);
}
public void add(Shape component) {
children.add(component);
}
public void add(Shape... components) {
children.addAll(Arrays.asList(components));
}
public void remove(Shape child) {
children.remove(child);
}
public void remove(Shape... components) {
children.removeAll(Arrays.asList(components));
}
public void clear() {
children.clear();
}
@Override
public int getX() {
if (children.size() == 0) {
return 0;
}
int x = children.get(0).getX();
for (Shape child : children) {
if (child.getX() < x) {
x = child.getX();
}
}
return x;
}
@Override
public int getY() {
if (children.size() == 0) {
return 0;
}
int y = children.get(0).getY();
for (Shape child : children) {
if (child.getY() < y) {
y = child.getY();
}
}
return y;
}
@Override
public int getWidth() {
int maxWidth = 0;
int x = getX();
for (Shape child : children) {
int childsRelativeX = child.getX() - x;
int childWidth = childsRelativeX + child.getWidth();
if (childWidth > maxWidth) {
maxWidth = childWidth;
}
}
return maxWidth;
}
@Override
public int getHeight() {
int maxHeight = 0;
int y = getY();
for (Shape child : children) {
int childsRelativeY = child.getY() - y;
int childHeight = childsRelativeY + child.getHeight();
if (childHeight > maxHeight) {
maxHeight = childHeight;
}
}
return maxHeight;
}
@Override
public void drag() {
for (Shape child : children) {
child.drag();
}
}
@Override
public void drop() {
for (Shape child : children) {
child.drop();
}
}
@Override
public void moveTo(int x, int y) {
for (Shape child : children) {
child.moveTo(x, y);
}
}
@Override
public void moveBy(int x, int y) {
for (Shape child : children) {
child.moveBy(x, y);
}
}
@Override
public boolean isInsideBounds(int x, int y) {
for (Shape child : children) {
if (child.isInsideBounds(x, y)) {
return true;
}
}
return false;
}
@Override
public void setColor(Color color) {
super.setColor(color);
for (Shape child : children) {
child.setColor(color);
}
}
@Override
public void unSelect() {
super.unSelect();
for (Shape child : children) {
child.unSelect();
}
}
public Shape getChildAt(int x, int y) {
for (Shape child : children) {
if (child.isInsideBounds(x, y)) {
return child;
}
}
return null;
}
public boolean selectChildAt(int x, int y) {
Shape child = getChildAt(x,y);
if (child != null) {
child.select();
return true;
}
return false;
}
public List<Shape> getSelected() {
List<Shape> selected = new ArrayList<>();
for (Shape child : children) {
if (child.isSelected()) {
selected.add(child);
}
}
return selected;
}
@Override
public void paint(Graphics graphics) {
if (isSelected()) {
enableSelectionStyle(graphics);
graphics.drawRect(getX() - 1, getY() - 1, getWidth() + 1, getHeight() + 1);
disableSelectionStyle(graphics);
}
for (Shape child : children) {
child.paint(graphics);
}
}
}
Demo.java: 초기화 코드
package refactoring_guru.memento.example;
import refactoring_guru.memento.example.editor.Editor;
import refactoring_guru.memento.example.shapes.Circle;
import refactoring_guru.memento.example.shapes.CompoundShape;
import refactoring_guru.memento.example.shapes.Dot;
import refactoring_guru.memento.example.shapes.Rectangle;
import java.awt.*;
public class Demo {
public static void main(String[] args) {
Editor editor = new Editor();
editor.loadShapes(
new Circle(10, 10, 10, Color.BLUE),
new CompoundShape(
new Circle(110, 110, 50, Color.RED),
new Dot(160, 160, Color.RED)
),
new CompoundShape(
new Rectangle(250, 250, 100, 100, Color.GREEN),
new Dot(240, 240, Color.GREEN),
new Dot(240, 360, Color.GREEN),
new Dot(360, 360, Color.GREEN),
new Dot(360, 240, Color.GREEN)
)
);
}
}
OutputDemo.png: 스크린샷
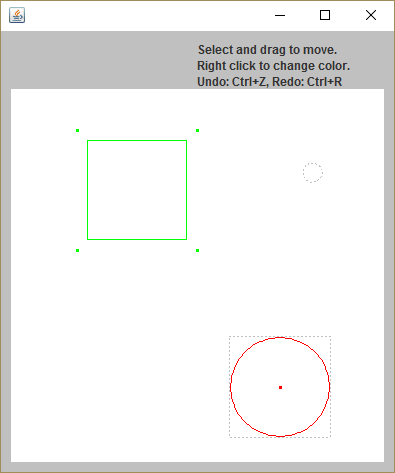