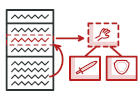
타입스크립트로 작성된 전략
전략은 행동들의 객체들을 객체들로 변환하며 이들이 원래 콘텍스트 객체 내에서 상호 교환이 가능하게 만드는 행동 디자인 패턴입니다.
원래 객체는 콘텍스트라고 불리며 전략 객체에 대한 참조를 포함합니다. 콘텍스트는 행동의 실행을 연결된 전략 객체에 위임합니다. 콘텍스트가 작업을 수행하는 방식을 변경하기 위하여 다른 객체들은 현재 연결된 전략 객체를 다른 전략 객체와 대체할 수 있습니다.
복잡도:
인기도:
사용 사례들: 전략 패턴은 타입스크립트 코드에서 매우 일반적으로 사용됩니다. 이 패턴은 다양한 프레임워크에서 사용자들이 클래스를 확장하지 않고 클래스의 행동을 변경할 수 있도록 자주 사용됩니다.
식별: 전략 패턴은 중첩된 객체가 실제 작업을 수행할 수 있도록 하는 메서드가 있으며 또 해당 객체를 다른 객체로 대체할 수 있는 세터가 있습니다.
개념적인 예시
이 예시는 전략 패턴의 구조를 보여주고 다음 질문에 중점을 둡니다:
- 패턴은 어떤 클래스들로 구성되어 있나요?
- 이 클래스들은 어떤 역할을 하나요?
- 패턴의 요소들은 어떻게 서로 연관되어 있나요?
index.ts: 개념적인 예시
/**
* The Context defines the interface of interest to clients.
*/
class Context {
/**
* @type {Strategy} The Context maintains a reference to one of the Strategy
* objects. The Context does not know the concrete class of a strategy. It
* should work with all strategies via the Strategy interface.
*/
private strategy: Strategy;
/**
* Usually, the Context accepts a strategy through the constructor, but also
* provides a setter to change it at runtime.
*/
constructor(strategy: Strategy) {
this.strategy = strategy;
}
/**
* Usually, the Context allows replacing a Strategy object at runtime.
*/
public setStrategy(strategy: Strategy) {
this.strategy = strategy;
}
/**
* The Context delegates some work to the Strategy object instead of
* implementing multiple versions of the algorithm on its own.
*/
public doSomeBusinessLogic(): void {
// ...
console.log('Context: Sorting data using the strategy (not sure how it\'ll do it)');
const result = this.strategy.doAlgorithm(['a', 'b', 'c', 'd', 'e']);
console.log(result.join(','));
// ...
}
}
/**
* The Strategy interface declares operations common to all supported versions
* of some algorithm.
*
* The Context uses this interface to call the algorithm defined by Concrete
* Strategies.
*/
interface Strategy {
doAlgorithm(data: string[]): string[];
}
/**
* Concrete Strategies implement the algorithm while following the base Strategy
* interface. The interface makes them interchangeable in the Context.
*/
class ConcreteStrategyA implements Strategy {
public doAlgorithm(data: string[]): string[] {
return data.sort();
}
}
class ConcreteStrategyB implements Strategy {
public doAlgorithm(data: string[]): string[] {
return data.reverse();
}
}
/**
* The client code picks a concrete strategy and passes it to the context. The
* client should be aware of the differences between strategies in order to make
* the right choice.
*/
const context = new Context(new ConcreteStrategyA());
console.log('Client: Strategy is set to normal sorting.');
context.doSomeBusinessLogic();
console.log('');
console.log('Client: Strategy is set to reverse sorting.');
context.setStrategy(new ConcreteStrategyB());
context.doSomeBusinessLogic();
Output.txt: 실행 결과
Client: Strategy is set to normal sorting.
Context: Sorting data using the strategy (not sure how it'll do it)
a,b,c,d,e
Client: Strategy is set to reverse sorting.
Context: Sorting data using the strategy (not sure how it'll do it)
e,d,c,b,a