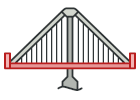
파이썬으로 작성된 브리지
브리지는 구조 디자인 패턴입니다. 이 패턴은 비즈니스 로직 또는 거대한 클래스를 독립적으로 개발할 수 있는 별도의 클래스 계층구조들로 나눕니다.
종종 추상화라고 불리는 이러한 계층구조 중 하나는 두 번째 계층구조의 객체(구현)에 대한 참조를 얻습니다. 추상화의 호출들 일부(때로는 대부분)를 구현 객체에 위임할 수 있습니다. 모든 구현은 공통 인터페이스를 가지므로 추상화 내에서 상호 교환할 수 있습니다.
복잡도:
인기도:
사용 예시들: 브리지 패턴은 크로스 플랫폼 앱들을 처리할 때, 여러 유형의 데이터베이스 서버를 지원할 때 또는 특정 종류의 여러 API 제공자(예: 클라우드 플랫폼, 소셜 네트워크 등)와 작업할 때 특히 유용합니다.
식별법: 브리지는 일부 제어 개체가 해당 개체가 의존하는 여러 다른 플랫폼들과 명확하게 구분됩니다.
개념적인 예시
이 예시는 브리지 디자인 패턴의 구조를 보여주고 다음 질문에 중점을 둡니다:
- 패턴은 어떤 클래스들로 구성되어 있나요?
- 이 클래스들은 어떤 역할을 하나요?
- 패턴의 요소들은 어떻게 서로 연관되어 있나요?
main.py: 개념적인 예시
from __future__ import annotations
from abc import ABC, abstractmethod
class Abstraction:
"""
The Abstraction defines the interface for the "control" part of the two
class hierarchies. It maintains a reference to an object of the
Implementation hierarchy and delegates all of the real work to this object.
"""
def __init__(self, implementation: Implementation) -> None:
self.implementation = implementation
def operation(self) -> str:
return (f"Abstraction: Base operation with:\n"
f"{self.implementation.operation_implementation()}")
class ExtendedAbstraction(Abstraction):
"""
You can extend the Abstraction without changing the Implementation classes.
"""
def operation(self) -> str:
return (f"ExtendedAbstraction: Extended operation with:\n"
f"{self.implementation.operation_implementation()}")
class Implementation(ABC):
"""
The Implementation defines the interface for all implementation classes. It
doesn't have to match the Abstraction's interface. In fact, the two
interfaces can be entirely different. Typically the Implementation interface
provides only primitive operations, while the Abstraction defines higher-
level operations based on those primitives.
"""
@abstractmethod
def operation_implementation(self) -> str:
pass
"""
Each Concrete Implementation corresponds to a specific platform and implements
the Implementation interface using that platform's API.
"""
class ConcreteImplementationA(Implementation):
def operation_implementation(self) -> str:
return "ConcreteImplementationA: Here's the result on the platform A."
class ConcreteImplementationB(Implementation):
def operation_implementation(self) -> str:
return "ConcreteImplementationB: Here's the result on the platform B."
def client_code(abstraction: Abstraction) -> None:
"""
Except for the initialization phase, where an Abstraction object gets linked
with a specific Implementation object, the client code should only depend on
the Abstraction class. This way the client code can support any abstraction-
implementation combination.
"""
# ...
print(abstraction.operation(), end="")
# ...
if __name__ == "__main__":
"""
The client code should be able to work with any pre-configured abstraction-
implementation combination.
"""
implementation = ConcreteImplementationA()
abstraction = Abstraction(implementation)
client_code(abstraction)
print("\n")
implementation = ConcreteImplementationB()
abstraction = ExtendedAbstraction(implementation)
client_code(abstraction)
Output.txt: 실행 결과
Abstraction: Base operation with:
ConcreteImplementationA: Here's the result on the platform A.
ExtendedAbstraction: Extended operation with:
ConcreteImplementationB: Here's the result on the platform B.