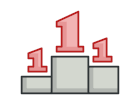
Singleton を TypeScript で
Singleton は、 生成に関するデザインパターンの一つで、 この種類のオブジェクトがただ一つだけ存在することを保証し、 他のコードに対して唯一のアクセス・ポイントを提供します。
Singleton には、 大域変数とほぼ同じ長所と短所があります。 両方とも随分と便利ですが、 コードのモジュール性を犠牲にしています。
シングルトンのクラスに依存しているあるクラスを使う場合、 シングルトンのクラスも一緒に使う必要があります。 ほとんどの場合、 この制限は、 ユニット・テストの作成で問題となります。
複雑度:
人気度:
使用例: 多くの開発者は、 Singleton をアンチ・パターンと見なしています。 TypeScript コードでの使用が減少したのはこのためです。
見つけ方: Singleton は、 キャッシュされた同一オブジェクトを返す静的生成メソッドで識別できます。
概念的な例
この例は、 Singleton デザインパターンの構造を説明するためのものです。 以下の質問に答えることを目的としています:
- どういうクラスからできているか?
- それぞれのクラスの役割は?
- パターンの要素同士はどう関係しているのか?
index.ts: 概念的な例
/**
* The Singleton class defines an `instance` getter, that lets clients access
* the unique singleton instance.
*/
class Singleton {
static #instance: Singleton;
/**
* The Singleton's constructor should always be private to prevent direct
* construction calls with the `new` operator.
*/
private constructor() { }
/**
* The static getter that controls access to the singleton instance.
*
* This implementation allows you to extend the Singleton class while
* keeping just one instance of each subclass around.
*/
public static get instance(): Singleton {
if (!Singleton.#instance) {
Singleton.#instance = new Singleton();
}
return Singleton.#instance;
}
/**
* Finally, any singleton can define some business logic, which can be
* executed on its instance.
*/
public someBusinessLogic() {
// ...
}
}
/**
* The client code.
*/
function clientCode() {
const s1 = Singleton.instance;
const s2 = Singleton.instance;
if (s1 === s2) {
console.log(
'Singleton works, both variables contain the same instance.'
);
} else {
console.log('Singleton failed, variables contain different instances.');
}
}
clientCode();
Output.txt: 実行結果
Singleton works, both variables contain the same instance.