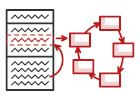
State em C#
O State é um padrão de projeto comportamental que permite que um objeto altere o comportamento quando seu estado interno for alterado.
O padrão extrai comportamentos relacionados ao estado em classes separadas de estado e força o objeto original a delegar o trabalho para uma instância dessas classes, em vez de agir por conta própria.
Complexidade:
Popularidade:
Exemplos de uso: O padrão State é comumente usado em C# para converter enormes máquinas de estado baseadas no switch
para dentro dos objetos.
Identificação: O padrão State pode ser reconhecido por métodos que alteram seu comportamento, dependendo do estado dos objetos, controlados externamente.
Exemplo conceitual
Este exemplo ilustra a estrutura do padrão de projeto State. Ele se concentra em responder a estas perguntas:
- De quais classes ele consiste?
- Quais papéis essas classes desempenham?
- De que maneira os elementos do padrão estão relacionados?
Program.cs: Exemplo conceitual
using System;
namespace RefactoringGuru.DesignPatterns.State.Conceptual
{
// The Context defines the interface of interest to clients. It also
// maintains a reference to an instance of a State subclass, which
// represents the current state of the Context.
class Context
{
// A reference to the current state of the Context.
private State _state = null;
public Context(State state)
{
this.TransitionTo(state);
}
// The Context allows changing the State object at runtime.
public void TransitionTo(State state)
{
Console.WriteLine($"Context: Transition to {state.GetType().Name}.");
this._state = state;
this._state.SetContext(this);
}
// The Context delegates part of its behavior to the current State
// object.
public void Request1()
{
this._state.Handle1();
}
public void Request2()
{
this._state.Handle2();
}
}
// The base State class declares methods that all Concrete State should
// implement and also provides a backreference to the Context object,
// associated with the State. This backreference can be used by States to
// transition the Context to another State.
abstract class State
{
protected Context _context;
public void SetContext(Context context)
{
this._context = context;
}
public abstract void Handle1();
public abstract void Handle2();
}
// Concrete States implement various behaviors, associated with a state of
// the Context.
class ConcreteStateA : State
{
public override void Handle1()
{
Console.WriteLine("ConcreteStateA handles request1.");
Console.WriteLine("ConcreteStateA wants to change the state of the context.");
this._context.TransitionTo(new ConcreteStateB());
}
public override void Handle2()
{
Console.WriteLine("ConcreteStateA handles request2.");
}
}
class ConcreteStateB : State
{
public override void Handle1()
{
Console.Write("ConcreteStateB handles request1.");
}
public override void Handle2()
{
Console.WriteLine("ConcreteStateB handles request2.");
Console.WriteLine("ConcreteStateB wants to change the state of the context.");
this._context.TransitionTo(new ConcreteStateA());
}
}
class Program
{
static void Main(string[] args)
{
// The client code.
var context = new Context(new ConcreteStateA());
context.Request1();
context.Request2();
}
}
}
Output.txt: Resultados da execução
Context: Transition to ConcreteStateA.
ConcreteStateA handles request1.
ConcreteStateA wants to change the state of the context.
Context: Transition to ConcreteStateB.
ConcreteStateB handles request2.
ConcreteStateB wants to change the state of the context.
Context: Transition to ConcreteStateA.