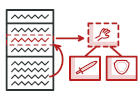
Strategia w języku Ruby
Strategia to behawioralny wzorzec projektowy zakładający przekształcenie zestawu zachowań w obiekty, które można stosować zamiennie w pierwotnym obiekcie.
Pierwotny obiekt, zwany kontekstem, przechowuje odniesienie do obiektu-strategii i deleguje mu działania związane z danym zachowaniem. Aby zmienić sposób, w jaki kontekst wykonuje swą pracę, należy zamienić bieżąco przypisany obiekt strategii na inny.
Złożoność:
Popularność:
Przykłady użycia: Wzorzec Strategia jest bardzo powszechny w kodzie Ruby. Często stosuje się go w różnych frameworkach by umożliwić użytkownikom zmianę funkcjonalności klasy bez konieczności rozszerzania jej.
Identyfikacja: Wzorzec Strategia można rozpoznać po obecności metody pozwalającej wykonywać faktyczną pracę zagnieżdżonemu obiektowi oraz po obecności settera umożliwiającego wymianę tego obiektu na inny.
Przykład koncepcyjny
Poniższy przykład ilustruje strukturę wzorca Strategia ze szczególnym naciskiem na następujące kwestie:
- Z jakich składa się klas?
- Jakie role pełnią te klasy?
- W jaki sposób elementy wzorca są ze sobą powiązane?
main.rb: Przykład koncepcyjny
# The Context defines the interface of interest to clients.
class Context
# The Context maintains a reference to one of the Strategy objects. The
# Context does not know the concrete class of a strategy. It should work with
# all strategies via the Strategy interface.
attr_writer :strategy
# Usually, the Context accepts a strategy through the constructor, but also
# provides a setter to change it at runtime.
def initialize(strategy)
@strategy = strategy
end
# Usually, the Context allows replacing a Strategy object at runtime.
def strategy=(strategy)
@strategy = strategy
end
# The Context delegates some work to the Strategy object instead of
# implementing multiple versions of the algorithm on its own.
def do_some_business_logic
# ...
puts 'Context: Sorting data using the strategy (not sure how it\'ll do it)'
result = @strategy.do_algorithm(%w[a b c d e])
print result.join(',')
# ...
end
end
# The Strategy interface declares operations common to all supported versions of
# some algorithm.
#
# The Context uses this interface to call the algorithm defined by Concrete
# Strategies.
class Strategy
# @abstract
#
# @param [Array] data
def do_algorithm(_data)
raise NotImplementedError, "#{self.class} has not implemented method '#{__method__}'"
end
end
# Concrete Strategies implement the algorithm while following the base Strategy
# interface. The interface makes them interchangeable in the Context.
class ConcreteStrategyA < Strategy
# @param [Array] data
#
# @return [Array]
def do_algorithm(data)
data.sort
end
end
class ConcreteStrategyB < Strategy
# @param [Array] data
#
# @return [Array]
def do_algorithm(data)
data.sort.reverse
end
end
# The client code picks a concrete strategy and passes it to the context. The
# client should be aware of the differences between strategies in order to make
# the right choice.
context = Context.new(ConcreteStrategyA.new)
puts 'Client: Strategy is set to normal sorting.'
context.do_some_business_logic
puts "\n\n"
puts 'Client: Strategy is set to reverse sorting.'
context.strategy = ConcreteStrategyB.new
context.do_some_business_logic
output.txt: Wynik działania
Client: Strategy is set to normal sorting.
Context: Sorting data using the strategy (not sure how it'll do it)
a,b,c,d,e
Client: Strategy is set to reverse sorting.
Context: Sorting data using the strategy (not sure how it'll do it)
e,d,c,b,a