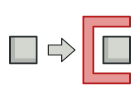
Pełnomocnik w języku Python
Pełnomocnik to strukturalny wzorzec projektowy według którego obiekt-usługodawca używany przez klienta jest zastępowany przez obiekt zastępczy, zwany pełnomocnikiem. Pełnomocnik przechwytuje żądania od klienta, wykonuje jakąś pracę (kontrola dostępu, zarządzanie pamięcią podręczną, itp.) a następnie przekazuje żądanie usługodawcy.
Obiekt będący pełnomocnikiem ma ten sam interfejs co usługodawca, co czyni go wymienialnym z obiektem usługodawcy dotychczas przekazywanym klientowi.
Złożoność:
Popularność:
Przykłady użycia: Pełnomocnik nie jest częstym gościem w aplikacjach napisanych w Pythonie, ale w niektórych wyjątkowych sytuacjach bardzo się przydaje. Jest niezastąpiony wszędzie tam, gdzie trzeba dodać jakąś funkcjonalność obiektowi istniejącej klasy bez zmiany kodu klienta.
Identyfikacja: Pełnomocnicy delegują całą faktyczną pracę innemu obiektowi. Każda metoda pełnomocnika powinna odnosić się do obiektu-usługodawcy, chyba, że pełnomocnik jest klasą pochodną usługi.
Przykład koncepcyjny
Poniższy przykład ilustruje strukturę wzorca Pełnomocnik ze szczególnym naciskiem na następujące kwestie:
- Z jakich składa się klas?
- Jakie role pełnią te klasy?
- W jaki sposób elementy wzorca są ze sobą powiązane?
main.py: Przykład koncepcyjny
from abc import ABC, abstractmethod
class Subject(ABC):
"""
The Subject interface declares common operations for both RealSubject and
the Proxy. As long as the client works with RealSubject using this
interface, you'll be able to pass it a proxy instead of a real subject.
"""
@abstractmethod
def request(self) -> None:
pass
class RealSubject(Subject):
"""
The RealSubject contains some core business logic. Usually, RealSubjects are
capable of doing some useful work which may also be very slow or sensitive -
e.g. correcting input data. A Proxy can solve these issues without any
changes to the RealSubject's code.
"""
def request(self) -> None:
print("RealSubject: Handling request.")
class Proxy(Subject):
"""
The Proxy has an interface identical to the RealSubject.
"""
def __init__(self, real_subject: RealSubject) -> None:
self._real_subject = real_subject
def request(self) -> None:
"""
The most common applications of the Proxy pattern are lazy loading,
caching, controlling the access, logging, etc. A Proxy can perform one
of these things and then, depending on the result, pass the execution to
the same method in a linked RealSubject object.
"""
if self.check_access():
self._real_subject.request()
self.log_access()
def check_access(self) -> bool:
print("Proxy: Checking access prior to firing a real request.")
return True
def log_access(self) -> None:
print("Proxy: Logging the time of request.", end="")
def client_code(subject: Subject) -> None:
"""
The client code is supposed to work with all objects (both subjects and
proxies) via the Subject interface in order to support both real subjects
and proxies. In real life, however, clients mostly work with their real
subjects directly. In this case, to implement the pattern more easily, you
can extend your proxy from the real subject's class.
"""
# ...
subject.request()
# ...
if __name__ == "__main__":
print("Client: Executing the client code with a real subject:")
real_subject = RealSubject()
client_code(real_subject)
print("")
print("Client: Executing the same client code with a proxy:")
proxy = Proxy(real_subject)
client_code(proxy)
Output.txt: Wynik działania
Client: Executing the client code with a real subject:
RealSubject: Handling request.
Client: Executing the same client code with a proxy:
Proxy: Checking access prior to firing a real request.
RealSubject: Handling request.
Proxy: Logging the time of request.