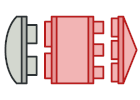
Адаптер на TypeScript
Адаптер — це структурний патерн, який дозволяє подружити несумісні об’єкти.
Адаптер виступає прошарком між двома об’єктами, перетворюючи виклики одного у виклики, що зрозумілі іншому.
Складність:
Популярність:
Застосування: Патерн можна часто зустріти в TypeScript-коді, особливо там, де потрібна конвертація різних типів даних або спільна робота класів з різними інтерфейсами.
Ознаки застосування патерна: Адаптер отримує конвертований об’єкт у конструкторі або через параметри своїх методів. Методи Адаптера, зазвичай, сумісні з інтерфейсом одного об’єкта. Вони делегують виклики вкладеному об’єктові, перетворивши перед цим параметри виклику у формат, підтримуваний вкладеним об’єктом.
Концептуальний приклад
Цей приклад показує структуру патерна Адаптер, а саме — з яких класів він складається, які ролі ці класи виконують і як вони взаємодіють один з одним.
index.ts: Приклад структури патерна
/**
* The Target defines the domain-specific interface used by the client code.
*/
class Target {
public request(): string {
return 'Target: The default target\'s behavior.';
}
}
/**
* The Adaptee contains some useful behavior, but its interface is incompatible
* with the existing client code. The Adaptee needs some adaptation before the
* client code can use it.
*/
class Adaptee {
public specificRequest(): string {
return '.eetpadA eht fo roivaheb laicepS';
}
}
/**
* The Adapter makes the Adaptee's interface compatible with the Target's
* interface.
*/
class Adapter extends Target {
private adaptee: Adaptee;
constructor(adaptee: Adaptee) {
super();
this.adaptee = adaptee;
}
public request(): string {
const result = this.adaptee.specificRequest().split('').reverse().join('');
return `Adapter: (TRANSLATED) ${result}`;
}
}
/**
* The client code supports all classes that follow the Target interface.
*/
function clientCode(target: Target) {
console.log(target.request());
}
console.log('Client: I can work just fine with the Target objects:');
const target = new Target();
clientCode(target);
console.log('');
const adaptee = new Adaptee();
console.log('Client: The Adaptee class has a weird interface. See, I don\'t understand it:');
console.log(`Adaptee: ${adaptee.specificRequest()}`);
console.log('');
console.log('Client: But I can work with it via the Adapter:');
const adapter = new Adapter(adaptee);
clientCode(adapter);
Output.txt: Результат виконання
Client: I can work just fine with the Target objects:
Target: The default target's behavior.
Client: The Adaptee class has a weird interface. See, I don't understand it:
Adaptee: .eetpadA eht fo roivaheb laicepS
Client: But I can work with it via the Adapter:
Adapter: (TRANSLATED) Special behavior of the Adaptee.