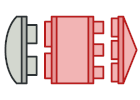
Adapter in Ruby
Adapter is a structural design pattern, which allows incompatible objects to collaborate.
The Adapter acts as a wrapper between two objects. It catches calls for one object and transforms them to format and interface recognizable by the second object.
Complexity:
Popularity:
Usage examples: The Adapter pattern is pretty common in Ruby code. It’s very often used in systems based on some legacy code. In such cases, Adapters make legacy code work with modern classes.
Identification: Adapter is recognizable by a constructor which takes an instance of a different abstract/interface type. When the adapter receives a call to any of its methods, it translates parameters to the appropriate format and then directs the call to one or several methods of the wrapped object.
Conceptual Example
This example illustrates the structure of the Adapter design pattern. It focuses on answering these questions:
- What classes does it consist of?
- What roles do these classes play?
- In what way the elements of the pattern are related?
main.rb: Conceptual example
# The Target defines the domain-specific interface used by the client code.
class Target
# @return [String]
def request
'Target: The default target\'s behavior.'
end
end
# The Adaptee contains some useful behavior, but its interface is incompatible
# with the existing client code. The Adaptee needs some adaptation before the
# client code can use it.
class Adaptee
# @return [String]
def specific_request
'.eetpadA eht fo roivaheb laicepS'
end
end
# The Adapter makes the Adaptee's interface compatible with the Target's
# interface.
class Adapter < Target
# @param [Adaptee] adaptee
def initialize(adaptee)
@adaptee = adaptee
end
def request
"Adapter: (TRANSLATED) #{@adaptee.specific_request.reverse!}"
end
end
# The client code supports all classes that follow the Target interface.
def client_code(target)
print target.request
end
puts 'Client: I can work just fine with the Target objects:'
target = Target.new
client_code(target)
puts "\n\n"
adaptee = Adaptee.new
puts 'Client: The Adaptee class has a weird interface. See, I don\'t understand it:'
puts "Adaptee: #{adaptee.specific_request}"
puts "\n"
puts 'Client: But I can work with it via the Adapter:'
adapter = Adapter.new(adaptee)
client_code(adapter)
output.txt: Execution result
Client: I can work just fine with the Target objects:
Target: The default target's behavior.
Client: The Adaptee class has a weird interface. See, I don't understand it:
Adaptee: .eetpadA eht fo roivaheb laicepS
Client: But I can work with it via the Adapter:
Adapter: (TRANSLATED) Special behavior of the Adaptee.