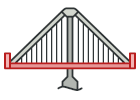
Most w języku TypeScript
Most jest strukturalnym wzorcem projektowym zakładającym podział logiki biznesowej lub dużej klasy na osobne hierarchie klas które następnie można rozwijać niezależnie od siebie.
Jedna z takich hierarchii (zwana często Abstrakcją) posiada referencję do obiektu drugiej hierarchii (zwanej Implementacją) i deleguje mu część (czasem większość) wywołań. Ponieważ wszystkie implementacje mają wspólny interfejs, z punktu widzenia abstrakcji są wymienialne.
Złożoność:
Popularność:
Przykłady użycia: Wzorzec Most jest szczególnie przydatny gdy trzeba wspierać obsługę wielu typów serwerów bazodanowych lub interfejsów programowania aplikacji danego typu (na przykład chmura, platformy społecznościowe, itd.)
Identyfikacja: Most można rozpoznać po wyraźnym rozdzieleniu na część kontrolującą i wiele różnych platform od których ta część zależy.
Przykład koncepcyjny
Poniższy przykład ilustruje strukturę wzorca Most ze szczególnym naciskiem na następujące kwestie:
- Z jakich składa się klas?
- Jakie role pełnią te klasy?
- W jaki sposób elementy wzorca są ze sobą powiązane?
index.ts: Przykład koncepcyjny
/**
* The Abstraction defines the interface for the "control" part of the two class
* hierarchies. It maintains a reference to an object of the Implementation
* hierarchy and delegates all of the real work to this object.
*/
class Abstraction {
protected implementation: Implementation;
constructor(implementation: Implementation) {
this.implementation = implementation;
}
public operation(): string {
const result = this.implementation.operationImplementation();
return `Abstraction: Base operation with:\n${result}`;
}
}
/**
* You can extend the Abstraction without changing the Implementation classes.
*/
class ExtendedAbstraction extends Abstraction {
public operation(): string {
const result = this.implementation.operationImplementation();
return `ExtendedAbstraction: Extended operation with:\n${result}`;
}
}
/**
* The Implementation defines the interface for all implementation classes. It
* doesn't have to match the Abstraction's interface. In fact, the two
* interfaces can be entirely different. Typically the Implementation interface
* provides only primitive operations, while the Abstraction defines higher-
* level operations based on those primitives.
*/
interface Implementation {
operationImplementation(): string;
}
/**
* Each Concrete Implementation corresponds to a specific platform and
* implements the Implementation interface using that platform's API.
*/
class ConcreteImplementationA implements Implementation {
public operationImplementation(): string {
return 'ConcreteImplementationA: Here\'s the result on the platform A.';
}
}
class ConcreteImplementationB implements Implementation {
public operationImplementation(): string {
return 'ConcreteImplementationB: Here\'s the result on the platform B.';
}
}
/**
* Except for the initialization phase, where an Abstraction object gets linked
* with a specific Implementation object, the client code should only depend on
* the Abstraction class. This way the client code can support any abstraction-
* implementation combination.
*/
function clientCode(abstraction: Abstraction) {
// ..
console.log(abstraction.operation());
// ..
}
/**
* The client code should be able to work with any pre-configured abstraction-
* implementation combination.
*/
let implementation = new ConcreteImplementationA();
let abstraction = new Abstraction(implementation);
clientCode(abstraction);
console.log('');
implementation = new ConcreteImplementationB();
abstraction = new ExtendedAbstraction(implementation);
clientCode(abstraction);
Output.txt: Wynik działania
Abstraction: Base operation with:
ConcreteImplementationA: Here's the result on the platform A.
ExtendedAbstraction: Extended operation with:
ConcreteImplementationB: Here's the result on the platform B.